C Exercises: Check three given integers and return true if the difference between small and medium and the difference between medium and large is same
33. Equal Difference Check
Write a C program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is the same.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int x, int y, int z);
int main(void) {
// Printing the results of calling 'test' function with different arguments
printf("%d", test(4, 5, 6));
printf("\n%d", test(7, 12, 13));
printf("\n%d", test(-1, 0, 1));
}
// Definition of the 'test' function
int test(int x, int y, int z) {
// Checking various conditions and returning the result of the comparisons
if (x > y && x > z && y > z) return x - y == y - z;
if (x > y && x > z && z > y) return x - z == z - y;
if (y > x && y > z && x > z) return y - x == x - z;
if (y > x && y > z && z > x) return y - z == z - x;
if (z > x && z > y && x > y) return z - x == x - y;
// If none of the above conditions are met, return the result of the final comparison
return z - y == y - x;
}
Sample Output:
1 0 1
Pictorial Presentation:
Flowchart:
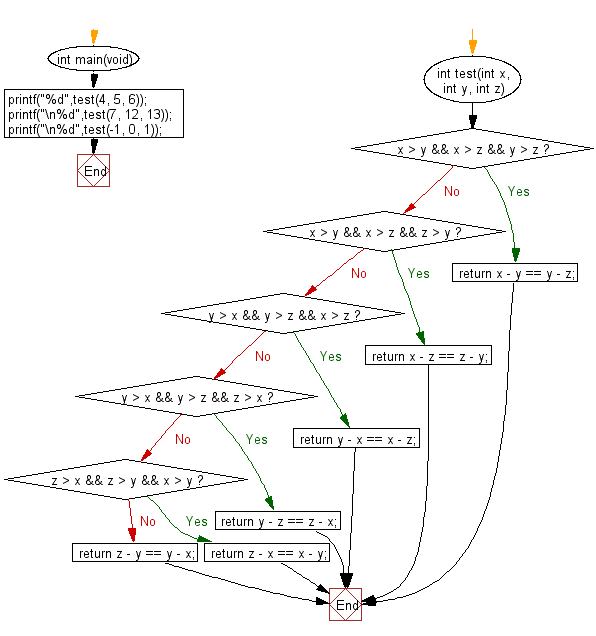
For more Practice: Solve these Related Problems:
- Write a C program to check if three numbers form an arithmetic sequence.
- Write a C program to verify if three integers are evenly spaced, allowing for a common difference of zero.
- Write a C program to determine if three given numbers have the same difference when sorted in ascending order.
- Write a C program to check if the difference between the first and second number is double the difference between the second and third.
C Programming Code Editor:
Previous: Write a C program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
Next: Write a C program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.