C Exercises: Check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array
34. Array Ends Equality Check
Write a C program to check a given array of integers of length 1 or more. Return true if the first element and the last element in the array are equal.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums[], int arr_size);
int main(void){
// Declaring variables
int arr_size;
// First test case with an array of integers
int array1[] = {1, 1, 2, 2, 1};
arr_size = sizeof(array1)/sizeof(array1[0]);
printf("%d",test(array1, arr_size));
// Second test case with a different array of integers
int array2[] = {1, 1, 2, 1, 2, 3};
arr_size = sizeof(array2)/sizeof(array2[0]);
printf("\n%d",test(array2, arr_size));
// Third test case with another array of integers
int array3[] = { 12, 24, 35, 55 };
arr_size = sizeof(array3)/sizeof(array3[0]);
printf("\n%d",test(array3, arr_size));
}
// Definition of the 'test' function
int test(int nums[], int arr_size)
{
// Checking if the array has at least one element and if the first and last elements are equal
return arr_size > 0 && nums[0] == nums[arr_size - 1];
}
Sample Output:
1 0 0
Pictorial Presentation:
Flowchart:
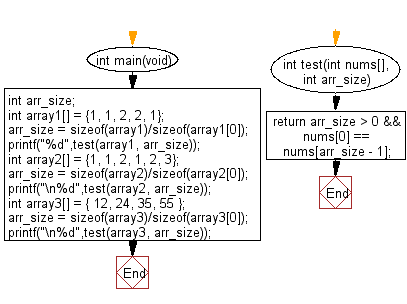
For more Practice: Solve these Related Problems:
- Write a C program to check if the first two and last two elements of an array are identical.
- Write a C program to verify if an array's first element is equal to its middle element in an odd-length array.
- Write a C program to determine if an array of integers has the same first and second-to-last element.
- Write a C program to check if both the first and last elements are prime numbers.
C Programming Code Editor:
Previous: Write a C program to check three given integers (small, medium and large) and return true if the difference between small and medium and the difference between medium and large is same.
Next: Write a C program to check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.