C Exercises: Check two given arrays of integers of length 1 or more and return true if they have the same first element or they have the same last element
35. Array First or Last Element Comparison
Write a C program to check two given arrays of integers of length 1 or more. Return true if they have the same first element or if they have the same last element.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int nums1[], int nums2[], int arr_size1, int arr_size2);
int main(void){
// Test case 1
int array11[] = {10, 20, 40, 50};
int arr_size11 = sizeof(array11)/sizeof(array11[0]);
int array12[] = {10, 20, 40, 50};
int arr_size12 = sizeof(array12)/sizeof(array12[0]);
printf("%d",test(array11,array12,arr_size11,arr_size12));
// Test case 2
int array21[] = {10, 20, 40, 50};
int arr_size21 = sizeof(array21)/sizeof(array21[0]);
int array22[] = {11, 20, 40, 51};
int arr_size22 = sizeof(array22)/sizeof(array22[0]);
printf("\n%d",test(array21,array22,arr_size21,arr_size22));
}
// Definition of the 'test' function
int test(int nums1[], int nums2[], int arr_size1, int arr_size2)
{
// Checking if the first element of the first array is equal to the first element of the second array
// or if the last element of the first array is equal to the last element of the second array
return nums1[0] == nums2[0] || nums1[arr_size1 - 1] == nums2[arr_size2 - 1];
}
Sample Output:
1 0
Pictorial Presentation:
Flowchart:
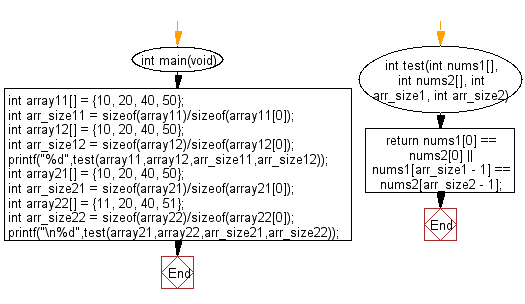
For more Practice: Solve these Related Problems:
- Write a C program to check if two arrays have at least one matching element at the same index.
- Write a C program to determine if two arrays share the same middle element in odd-length arrays.
- Write a C program to verify if two arrays have either the same first element or share a common element anywhere.
- Write a C program to check if the first element of one array matches the last element of another array.
C Programming Code Editor:
Previous: Write a C program to check a given array of integers of length 1 or more and return true if the first element and the last element are equal in the given array.
Next: Write a C program to compute the sum of the elements of an given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.