C Exercises: Compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17
C-programming basic algorithm: Exercise-31 with Solution
Write a C program to compute the sum of the three given integers with some exceptions. If any of the values is in the range 10..20 inclusive, then that value will be considered as 0, except for 13 and 17.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototypes for 'test' and 'fix_num'
int test(int x, int y, int z);
int fix_num(int n);
int main(void){
// Printing the results of calling 'test' function with different arguments
printf("%d",test(4, 5, 7));
printf("\n%d",test(7, 4, 12));
printf("\n%d",test(10, 13, 12));
printf("\n%d",test(13, 12, 18));
}
// Definition of the 'test' function
int test(int x, int y, int z)
{
// Calling 'fix_num' function on each input and returning their sum
return fix_num(x) + fix_num(y) + fix_num(z);
}
// Definition of the 'fix_num' function
int fix_num(int n)
{
// Checking if 'n' is in the range (10, 13) or (18, 20) and returning 0 if true, otherwise returning 'n'
return (n < 13 && n > 9) || (n > 17 && n < 21) ? 0 : n;
}
Sample Output:
16 11 13 13
Pictorial Presentation:
Flowchart:
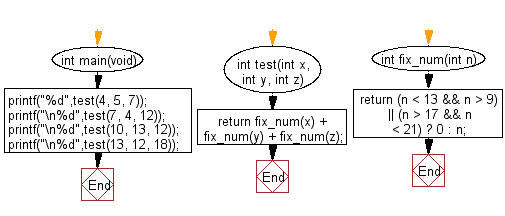
C Programming Code Editor:
Previous: Write a C program to compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum.
Next: Write a C program to check two given integers and return the value whichever value is nearest to 13 without going over. Return 0 if both numbers go over.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-31.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics