C Exercises: Compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum
C-programming basic algorithm: Exercise-30 with Solution
Write a C program to compute the sum of the three integers. Do not count a value that is 13 and add it to the sum.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int x, int y, int z);
int main(void){
// Printing the results of calling 'test' function with different arguments
printf("%d",test(4, 5, 7));
printf("\n%d",test(7, 4, 12));
printf("\n%d",test(10, 13, 12));
printf("\n%d",test(13, 12, 18));
}
// Definition of the 'test' function
int test(int x, int y, int z)
{
// Checking if 'x' is equal to 13
if (x == 13) return 0;
// Checking if 'y' is equal to 13
if (y == 13) return x;
// Checking if 'z' is equal to 13
if (z == 13) return y + z;
// If none of the above conditions are met, return the sum of all three arguments
return x + y + z;
}
Sample Output:
16 23 10 0
Explanation:
int test(int x, int y, int z) { if (x == 13) return 0; if (y == 13) return x; if (z == 13) return y + z; return x + y + z; }
The function starts by checking if the first integer argument is equal to 13. If it is, then the function returns 0, as specified.
If the first argument is not equal to 13, then the function proceeds to check the second argument.
If the second argument is equal to 13, then the function returns the first argument (excluding the second argument from the sum).
If the second argument is not equal to 13, then the function proceeds to check the third argument.
If the third argument is equal to 13, then the function returns the sum of the first and second arguments (excluding the third argument from the sum).
If none of the arguments are equal to 13, then the function returns the sum of all three arguments.
Time complexity and space complexity:
The time complexity of the function is O(1) as the function has a constant time complexity since it performs a fixed number of comparisons and arithmetic operations that are not dependent on the size of the input.
The space complexity of the function is O(1) as the function uses a constant amount of memory since it only uses a few integer variables to store the input values and the intermediate results.
Pictorial Presentation:
Flowchart:
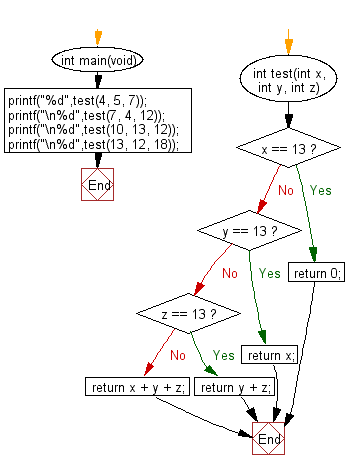
C Programming Code Editor:
Previous: Write a C program to compute the sum of three given integers. If the two values are same return the third value.
Next: Write a C program to compute the sum of the three given integers. However, if any of the values is in the range 10..20 inclusive then that value counts as 0, except 13 and 17.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics