C Exercises: Compute the sum of three given integers. If the two values are same return the third value
29. Sum of Three with Duplicate Check
Write a C program to compute the sum of three given integers. Return the third value if the two values are the same.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'test'
int test(int x, int y, int z);
int main(void){
// Printing the results of calling 'test' function with different arguments
printf("%d",test(4, 5, 7));
printf("\n%d",test(7, 4, 12));
printf("\n%d",test(10, 10, 12));
printf("\n%d",test(12, 12, 18));
}
// Definition of the 'test' function
int test(int x, int y, int z)
{
// Checking if all three arguments are equal
if (x == y && y == z) return 0;
// Checking if 'x' and 'y' are equal
if (x == y) return z;
// Checking if 'x' and 'z' are equal
if (x == z) return y;
// Checking if 'y' and 'z' are equal
if (y == z) return x;
// If none of the above conditions are met, return the sum of all three arguments
return x + y + z;
}
Sample Output:
16 23 12 18
Explanation:
int test(int x, int y, int z) { if (x == y && y == z) return 0; if (x == y) return z; if (x == z) return y; if (y == z) return x; return x + y + z; }
The above function first checks if all three integers are the same, in which case it returns 0. If not, it checks for each pair of integers to see if they are the same. If a pair is found, the function returns the value of the third integer. If none of the pairs are equal, the function returns the sum of all three integers.
Time complexity and space complexity:
The time complexity of the function is O(1) as it performs a constant number of comparisons and arithmetic operations, so its time complexity is constant.
The space complexity of the function is O(1) as it uses a constant amount of memory, regardless of the input size.
Pictorial Presentation:
Flowchart:
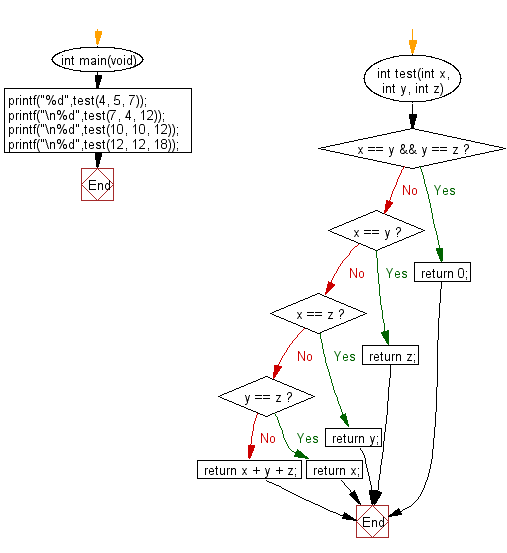
For more Practice: Solve these Related Problems:
- Write a C program to compute the product of three numbers, but if the first two are equal, return the third number instead.
- Write a C program to sum three integers, but return zero if the first two numbers are equal.
- Write a C program to add three integers, and if the first two are the same, return double the third value.
- Write a C program to compute the sum of three integers, and if any two are equal, subtract the third from the sum.
C Programming Code Editor:
Previous: Write a C program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33.
Next: Write a C program to compute the sum of the three integers. If one of the values is 13 then do not count it and its right towards the sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.