C Exercises: Check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33
28. Common Digit in Two Two-Digit Numbers
Write a C program to check two given integers. Each integer is in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
int main(void){
printf("%d",test(11, 21)); // Call the 'test' function with arguments 11 and 21 and print the result
printf("\n%d",test(11, 20)); // Call the 'test' function with arguments 11 and 20 and print the result
printf("\n%d",test(10, 10)); // Call the 'test' function with arguments 10 and 10 and print the result
}
int test(int x, int y) // Define the 'test' function that takes two integers 'x' and 'y'
{
// Return true if any of the following conditions are met:
// 1. x/10 equals y/10 (both have the same tens digit)
// 2. x/10 equals y%10 (tens digit of x equals units digit of y)
// 3. x%10 equals y/10 (units digit of x equals tens digit of y)
// 4. x%10 equals y%10 (both have the same units digit)
return x / 10 == y / 10 || x / 10 == y % 10 || x % 10 == y / 10 || x % 10 == y % 10;
}
Sample Output:
1 0 1
Explanation:
int test(int x, int y) { return x / 10 == y / 10 || x / 10 == y % 10 || x % 10 == y / 10 || x % 10 == y % 10; }
The above function takes two integer arguments, x and y. It checks whether any of the digits of x and y are the same.
- It first checks whether the tens digit of x and y are the same.
- Then check whether the tens digit of x is the same as the ones digit of y.
- Then check whether the ones digit of x is the same as the tens digit of y.
- Finally check whether the ones digit of x and y are the same.
If any of these conditions are true, it returns 1, otherwise, it returns 0.
Time complexity and space complexity:
The time complexity of the function is O(1) since it always performs a fixed number of operations regardless of the input values.
The space complexity of the function is O(1) as the function uses a constant amount of space since it only stores two integer variables and a few temporary variables used in the calculations.
Pictorial Presentation:
Flowchart:
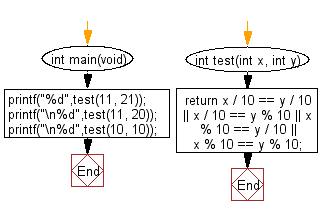
For more Practice: Solve these Related Problems:
- Write a C program to check if two two-digit numbers share any common digit, regardless of order.
- Write a C program to verify if any digit from the first two-digit number appears in the second one in reverse order.
- Write a C program that returns true if two given integers (10-99) have a sum of their digits that is identical.
- Write a C program to determine if the tens digit of one number matches the units digit of another.
C Programming Code Editor:
Previous: Write a C program to find the larger from two given integers. However if the two integers have the same remainder when divided by 5, then the return the smaller integer. If the two integers are the same, return 0.
Next: Write a C program to compute the sum of three given integers. If the two values are same return the third value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.