C Exercises: Find the larger from two given integers of specified pattern
27. Conditional Larger Integer with Remainder Check
Write a C program to find the larger of two given integers. However if the two integers have the same remainder when divided by 5, choose the smaller integer. If the two integers are the same, return 0.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with two integer parameters 'x' and 'y'
int test(int x, int y);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(11, 21));
printf("\n%d",test(11, 20));
printf("\n%d",test(10, 10));
}
// Function definition for 'test'
int test(int x, int y)
{
if (x == y) // If 'x' is equal to 'y'
{
return 0; // Return 0
}
else if ((x % 5 == y % 5 && x < y) || x > y) // If the conditions inside the parentheses are met
{
return x; // Return 'x'
}
else
{
return y; // Return 'y'
}
}
Sample Output:
11 20 0
Explanation:
int test(int x, int y, int z) { if (x == y) { return 0; } else if ((x % 5 == y % 5 && x < y) || x > y) { return x; } else { return y; } }
The above function takes three integer inputs and returns an integer value. It first checks if the first and second input integers are equal. If they are equal, the function returns 0. Otherwise, it checks if the remainder of the first integer divided by 5 is equal to the remainder of the second integer divided by 5 and the first integer is less than the second integer. If this condition is true, then the function returns the value of the first integer. Otherwise, it returns the value of the second integer.
Time complexity and space complexity:
The time complexity of this function is O(1) because the execution time is not dependent on the input size.
The space complexity of this function is O(1) because the function uses only a constant amount of memory space irrespective of the input size.
Pictorial Presentation:
Flowchart:
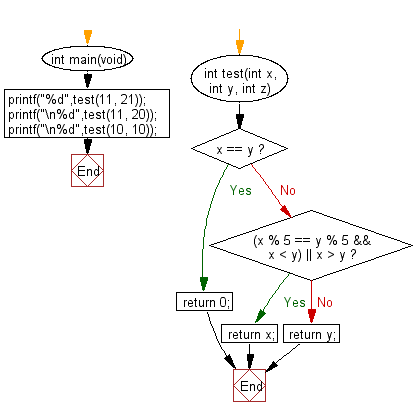
For more Practice: Solve these Related Problems:
- Write a C program to find the smaller of two numbers unless their difference is a multiple of 7, then return the larger.
- Write a C program that compares two integers, but if both are even, return the product of their remainders when divided by 3.
- Write a C program to determine the larger of two numbers, but if they have the same last digit, return their sum.
- Write a C program to compare two integers and return 0 if both have the same remainder when divided by 4, otherwise return the difference.
C Programming Code Editor:
Previous: Write a C program to check three given integers and return true if one of them is 20 or more less than one of the others.
Next: Write a C program to check two given integers, each in the range 10..99. Return true if a digit appears in both numbers, such as the 3 in 13 and 33
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.