C Exercises: Check three given integers and return true if one of them is 20 or more less than one of the others
26. Comparative Check with Threshold 20
Write a C program to check three given integers and return true if one of them is 20 or more and less than one of the others.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with three integer parameters 'x', 'y', and 'z'
int test(int x, int y, int z);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(11, 21, 31));
printf("\n%d",test(11, 22, 31));
printf("\n%d",test(10, 20, 15));
}
// Function definition for 'test'
int test(int x, int y, int z)
{
// Check if the absolute difference between 'x' and 'y' is greater than or equal to 20
// or the absolute difference between 'x' and 'z' is greater than or equal to 20
// or the absolute difference between 'y' and 'z' is greater than or equal to 20
if (abs(x - y) >= 20 || abs(x - z) >= 20 || abs(y - z) >= 20) {
return 1; // If any of these conditions is true, return 1
} else {
return 0; // If none of the conditions is true, return 0
}
}
Sample Output:
1 1 0
Explanation:
int test(int x, int y, int z) { return abs(x - y) >= 20 || abs(x - z) >= 20 || abs(y - z) >= 20; }
The function ‘test’ takes in three integers x, y, and z. First, the function checks if the absolute difference between x and y is greater than or equal to 20, and if so, returns 1. Then, it checks if the absolute difference between x and z is greater than or equal to 20, and if so, returns 1. Finally, it checks if the absolute difference between y and z is greater than or equal to 20, and if so, returns 1. If none of these conditions are met, it returns 0.
Time complexity and space complexity:
The time complexity of the function is O(1), as it only performs a fixed number of operations, regardless of the input size.
The space complexity of the function is O(1), as it only uses a fixed amount of memory to store the input variables and any local variables.
Pictorial Presentation:
Flowchart:
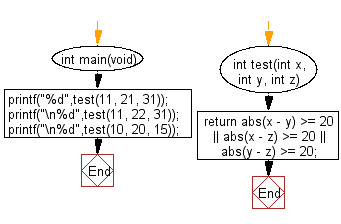
For more Practice: Solve these Related Problems:
- Write a C program that checks if one of three integers is at least 30 and less than another integer.
- Write a C program to verify if any one of three numbers falls strictly between the other two.
- Write a C program to determine if the smallest of three numbers is at least 15 and less than the largest.
- Write a C program to check if any of three numbers is between 20 and 40, while the others are outside this range.
C Programming Code Editor:
Previous: Write a C program to check if two or more non-negative given integers have the same rightmost digit.
Next: Write a C program to find the larger from two given integers. However if the two integers have the same remainder when divided by 5, then the return the smaller integer. If the two integers are the same, return 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.