C Exercises: Check whether two or more non-negative given integers have the same rightmost digit
C-programming basic algorithm: Exercise-25 with Solution
Write a C program to check if two or more nonnegative integers have the same rightmost digit.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with three integer parameters 'x', 'y', and 'z'
int test(int x, int y, int z);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(11, 21, 31));
printf("\n%d",test(11, 22, 31));
printf("\n%d",test(11, 22, 33));
}
// Function definition for 'test'
int test(int x, int y, int z)
{
// Check if the last digit of 'x' is equal to the last digit of 'y' or 'z'
// or if the last digit of 'y' is equal to the last digit of 'z'
if (x % 10 == y % 10 || x % 10 == z % 10 || y % 10 == z % 10) {
return 1; // If any of these conditions is true, return 1
} else {
return 0; // If none of the conditions is true, return 0
}
}
Sample Output:
1 1 0
Explanation:
int test(int x, int y, int z) { return x % 10 == y % 10 || x % 10 == z % 10 || y % 10 == z % 10; }
The function uses the modulo operator (%) to get the last digit of each integer by taking the remainder when it is divided by 10. It then compares the last digits of all possible pairs of integers to see if any two have the same last digit. If at least two integers have the same last digit, it returns 1, otherwise it returns 0.
Time complexity and space complexity:
The time complexity of this function is O(1) because it performs a constant number of operations regardless of the values of the input parameters.
The space complexity of this function is O(1) because it uses a constant amount of memory to store the integer parameters and local variables.
Pictorial Presentation:
Flowchart:
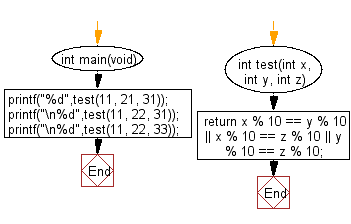
C Programming Code Editor:
Previous: Write a C program to check if y is greater than x, and z is greater than y from three given integers x,y,z.
Next: Write a C program to check three given integers and return true if one of them is 20 or more less than one of the others.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-25.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics