C Exercises: Check whether y is greater than x, and z is greater than y from three given integers x,y,z
C-programming basic algorithm: Exercise-24 with Solution
Write a C program that checks if y is greater than x and z is greater than y, given three integers x, y, and z. If both conditions are true, the program returns true. Otherwise, it returns false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with three integer parameters 'x', 'y', and 'z'
int test(int x, int y, int z);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(1, 2, 3));
printf("\n%d",test(4, 5, 6));
printf("\n%d",test(-1, 1, 0));
}
// Function definition for 'test'
int test(int x, int y, int z)
{
// Check if 'x' is less than 'y' and 'y' is less than 'z'
if (x < y && y < z) {
return 1; // If the condition is true, return 1
} else {
return 0; // If the condition is false, return 0
}
}
Sample Output:
1 1 0
Explanation:
int test(int x, int y, int z) { return x < y && y < z; }
The function takes three integer arguments, x, y, and z. It checks whether x is less than y and y is less than z. If both conditions are true, it returns 1; otherwise, it returns 0.
Time complexity and space complexity:
The time complexity of this function is O(1), as the execution time of the function does not depend on the size of the input.
The space complexity of this function is also O(1), as it uses only a constant amount of additional space regardless of the size of the input.
Pictorial Presentation:
Flowchart:
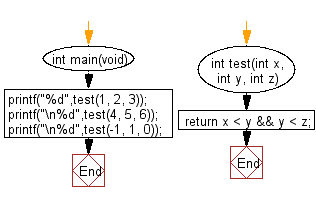
C Programming Code Editor:
Previous: Write a C program to check whether it is possible to add two integers to get the third integer from three given integers.
Next: Write a C program to check whether two or more non-negative given integers have the same rightmost digit
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics