C Exercises: Check whether it is possible to add two integers to get the third integer from three given integers
C-programming basic algorithm: Exercise-23 with Solution
Write a C program to check whether it is possible to add two integers to get the third integer from three given integers.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with three integer parameters 'x', 'y', and 'z'
int test(int x, int y, int z);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(1, 2, 3));
printf("\n%d",test(4, 5, 6));
printf("\n%d",test(-1, 1, 0));
}
// Function definition for 'test'
int test(int x, int y, int z)
{
// Check if any two numbers among 'x', 'y', and 'z' add up to the third number
if (x == y + z || y == x + z || z == x + y) {
return 1; // If the condition is true, return 1
} else {
return 0; // If the condition is false, return 0
}
}
Sample Output:
1 0 1
Explanation:
int test(int x, int y, int z) { return x == y + z || y == x + z || z == x + y; }
The function takes three integers x, y, and z as input and checks if any two of them add up to the third one. It returns 1 if the condition is true, otherwise, it returns 0.
Time complexity and space complexity:
The time complexity of the given function is O(n) as it performs a fixed number of operations irrespective of the size of the inputs.
Space complexity: O(1)
The space complexity of the given function is O(1) as the function uses a fixed amount of memory to store the input variables and the return value. Therefore, the space complexity is constant.
Pictorial Presentation:
Flowchart:
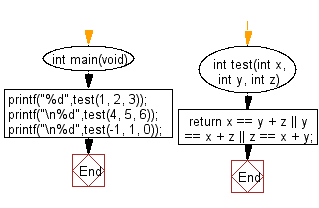
C Programming Code Editor:
Previous: Write a C program to compute the sum of the two given integers. If one of the given integer value is in the range 10..20 inclusive return 18.
Next: Write a C program to check whether y is greater than x, and z is greater than y from three given integers x,y,z.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics