C Exercises: Compute the sum of the two specified integers. If one of the given integer value is in the range 10..20 inclusive return 18
22. Sum with Special Range Override
Write a C program to compute the sum of the two given integers. Return 18 if one of the integer values given is in the range 10..20 inclusive.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with two integer parameters 'x' and 'y'
int test(int x, int y);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(3, 7));
printf("\n%d",test(10, 11));
printf("\n%d",test(10, 20));
printf("\n%d",test(21, 220));
}
// Function definition for 'test'
int test(int x, int y)
{
// Check if either 'x' or 'y' is within the range of 10 to 20 (inclusive)
if (x >= 10 && x <= 20 || y >= 10 && y <= 20) {
return 18; // If the condition is true, return 18
} else {
return x + y; // If the condition is false, return the sum of 'x' and 'y'
}
}
Sample Output:
10 18 18 241
Explanation:
int test(int x, int y) { return (x >= 10 && x <= 20) || (y >= 10 && y <= 20) ? 18 : x + y; }
The function takes two integer arguments x and y, and returns 18 if either x or y is between 10 and 20 (inclusive). Otherwise, it returns the sum of x and y.
Time complexity and space complexity:
The time complexity of this function is O(1) because it only performs a constant number of operations, regardless of the input size.
The space complexity of this function is O(1) because it only uses a constant amount of memory to store the input arguments and the return value.
Pictorial Presentation:
Flowchart:
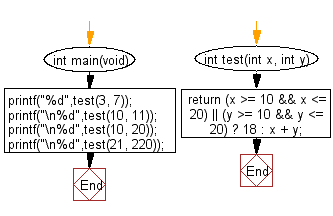
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of two integers, but return 25 if one of the integers is between 15 and 25 inclusive.
- Write a C program that adds two numbers and returns a fixed value if their sum is within a given range.
- Write a C program to sum two integers, but return triple the sum if one is in the range 5..15.
- Write a C program that computes the sum of two numbers and returns a constant if either number is within a specific range.
C Programming Code Editor:
Previous: Write a C program to check if a given number is within 2 of a multiple of 10.
Next: Write a C program to check if it is possible to add two integers to get the third integer from three given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.