C Exercises: Check whether a given number is within 2 of a multiple of 10
21. Near Multiple of 10 Check
Write a C program to check whether a given number is within 2 of a multiple of 10.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Function declaration for 'test' with an integer parameter 'n'
int test(int n);
int main(void){
// Print the result of calling 'test' with different integer values and format the output
printf("%d",test(3));
printf("\n%d",test(7));
printf("\n%d",test(8));
printf("\n%d",test(21));
}
// Function definition for 'test'
int test(int n)
{
// Check if the last digit of 'n' is less than or equal to 2 OR greater than or equal to 8
if (n % 10 <= 2 || n % 10 >= 8) {
return 1; // If the condition is true, return 1 (true)
} else {
return 0; // If the condition is false, return 0 (false)
}
}
Sample Output:
0 0 1 1
Explanation:
int test(int n) { return n % 10 <= 2 || n % 10 >= 8; }
The above function takes an integer n as input and returns 1 if the remainder of n divided by 10 is less than or equal to 2 or greater than or equal to 8, and 0 otherwise.
Time complexity and space complexity:
The time complexity of the function is O(1) as it performs a constant number of operations regardless of the input value.
The Space complexity of the function is O(1) as it uses a constant amount of space regardless of the input value.
Flowchart:
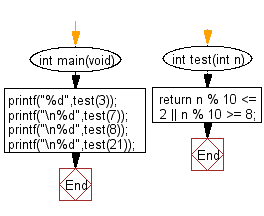
For more Practice: Solve these Related Problems:
- Write a C program to check if a given number is within 3 of a multiple of 15.
- Write a C program to verify if a number is within 1 of a multiple of 8.
- Write a C program that checks if an integer is within 2 of a multiple of 12.
- Write a C program to determine if a number is within 5 of a multiple of 20.
C Programming Code Editor:
Previous: Write a C program to check whether a given non-negative number is a multiple of 3 or 7, but not both.
Next: Write a C program compute the sum of the two given integers. If one of the given integer value is in the range 10..20 inclusive return 18.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.