C Exercises: Reverse a given array of integers and length 5
C-programming basic algorithm: Exercise-38 with Solution
Write a C program to reverse a given array of integers of length 5.
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function prototype for 'print_array'
void print_array(int parray[], int size);
int main(void){
// Declaration and initialization of variables
int arr_size;
int a1[] = {10, 20, 30, 40, 50};
// Calculating the size of the array
arr_size = sizeof(a1)/sizeof(a1[0]);
// Printing the original array
printf("Elements in original array are: ");
print_array(a1, arr_size);
// Creating a new array with elements in reverse order from the original array
int result[] = { a1[4], a1[3], a1[2], a1[1], a1[0] };
// Calculating the size of the new array
arr_size = sizeof(result)/sizeof(result[0]);
// Printing the reversed array
printf("\nElements in reverse array are: ");
print_array(result, arr_size);
}
// Definition of the 'print_array' function
void print_array(int parray[], int size)
{
int i;
for( i=0; i<size-1; i++)
{
// Printing each element with a comma and a space
printf("%d, ", parray[i]);
}
// Printing the last element without a comma and space
printf("%d ", parray[i]);
// Printing a new line to separate the elements
printf("\n");
}
Sample Output:
Elements in original array are: 10, 20, 30, 40, 50 Elements in reverse array are: 50, 40, 30, 20, 10
Pictorial Presentation:
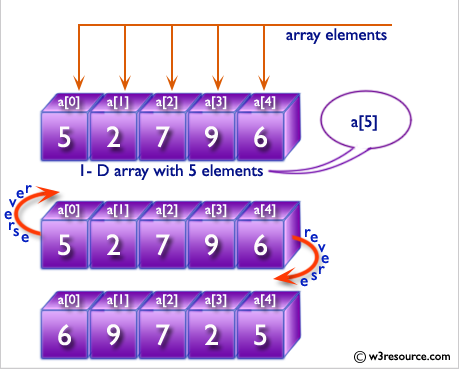
Flowchart:
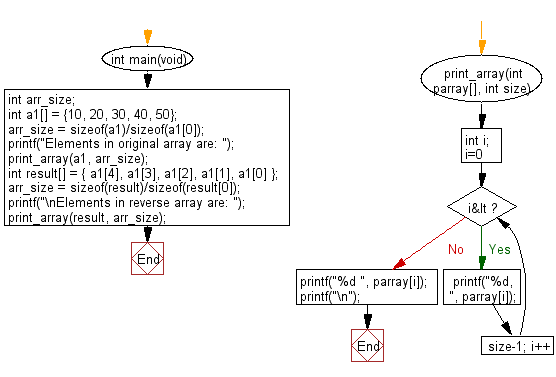
C Programming Code Editor:
Previous: Write a C program to rotate the elements of a given array of integers (length 4 )in left direction and return the new array.
Next: Write a C program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-38.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics