C Exercises: Sum or product of an array of integers
C Callback function: Exercise-3 with Solution
Write a C program to calculate the sum or product of an array of integers using a callback function.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
typedef int( * operation_func_t)(int, int);
int calculate(int * arr, size_t n, int initial_value, operation_func_t operation) {
int result = initial_value;
for (size_t i = 0; i < n; i++) {
result = operation(result, arr[i]);
}
return result;
}
int sum(int a, int b) {
return a + b;
}
int product(int a, int b) {
return a * b;
}
int main() {
int arr[] = {
10,
20,
30,
40,
50,
60
};
size_t n = sizeof(arr) / sizeof(int);
printf("Original array elements: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
int sum_result = calculate(arr, n, 0, sum);
printf("\nSum: %d", sum_result);
int product_result = calculate(arr, n, 1, product);
printf("\nProduct: %d", product_result);
return 0;
}
Sample Output:
Original array elements: 10 20 30 40 50 60 Sum: 210 Product: 720000000
Explanation:
In the above program, we define a function calculate() that takes an array of integers, its size, an initial value, and a callback function ‘operation’ as arguments. The ‘operation’ function takes two integers as arguments and returns the result of applying a mathematical operation to them, such as addition or multiplication. The calculate() function applies the operation function to each element of the array, accumulating the result in a variable result, starting with the initial_value.
We then define two callback functions sum() and product() that take two integers as arguments and return their sum and product, respectively.
In the main() function, we create an array of integers and call calculate() twice with different operation functions to calculate the sum and product of the array.
Flowchart:
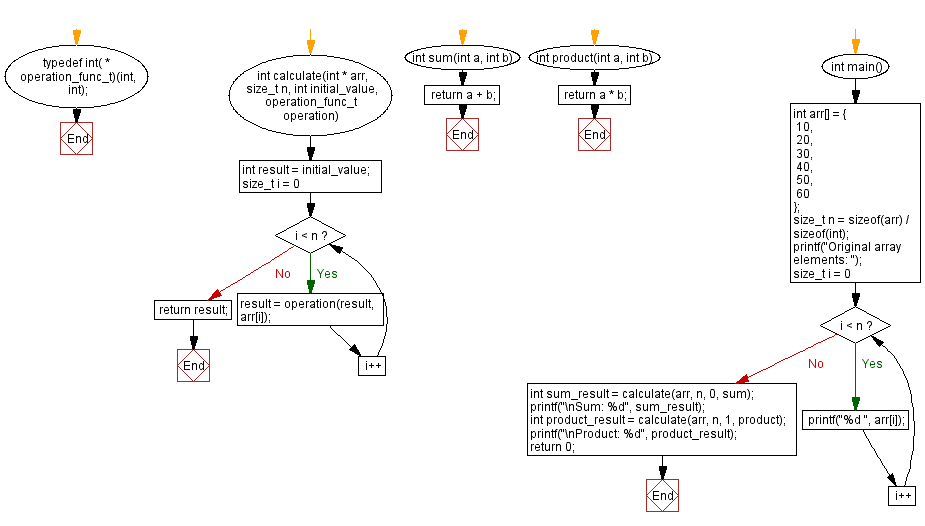
C Programming Code Editor:
Previous: Sort an array of integers.
Next: String is a palindrome or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics