C Exercises: Sort an array of integers
2. Array Sorting with Callback Variants
Write C program to sort an array of integers in ascending or descending order using a callback function to compare the elements.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
typedef int( * compare_func_t)(const void * ,
const void * );
void sort_array(int * arr, size_t n, compare_func_t compare) {
qsort(arr, n, sizeof(int), compare);
}
int ascending_order(const void * a,
const void * b) {
const int * ia = (const int * ) a;
const int * ib = (const int * ) b;
return ( * ia > * ib) - ( * ia < * ib);
}
int descending_order(const void * a,
const void * b) {
const int * ia = (const int * ) a;
const int * ib = (const int * ) b;
return ( * ib > * ia) - ( * ib < * ia);
}
int main() {
int arr[] = {
7,
2,
0,
5,
8,
9
};
size_t n = sizeof(arr) / sizeof(int);
printf("Original array: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
sort_array(arr, n, ascending_order);
printf("\nAscending order: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
sort_array(arr, n, descending_order);
printf("\nDescending order: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
Sample Output:
Original array: 7 2 0 5 8 9 Ascending order: 0 2 5 7 8 9 Descending order: 9 8 7 5 2 0
Explanation:
In the above program, we define a function sort_array() that takes an array (type integers), its size, and a callback function ‘compare’ as arguments. The ‘compare’ function is used to compare two elements of the array and return a negative value if the first element should come before the second, a positive value if the first element should come after the second, or zero if the elements are equal. Here we use the qsort() function from the standard library to perform the sorting, passing in the array, its size, the size of each element, and the compare function.
We then define two callback functions ascending_order() and descending_order(), which compare two integers and return a value indicating their order. The ascending_order() function returns a negative value if the first integer is less than the second, and a positive value if the first integer is greater than the second. The descending_order() function returns the opposite order, with a negative value if the first integer is greater than the second, and a positive value if the first integer is less than the second.
In the main() function, we create an array of integers and call sort_array() twice with different compare functions to sort the array in ascending and descending order.
Flowchart:
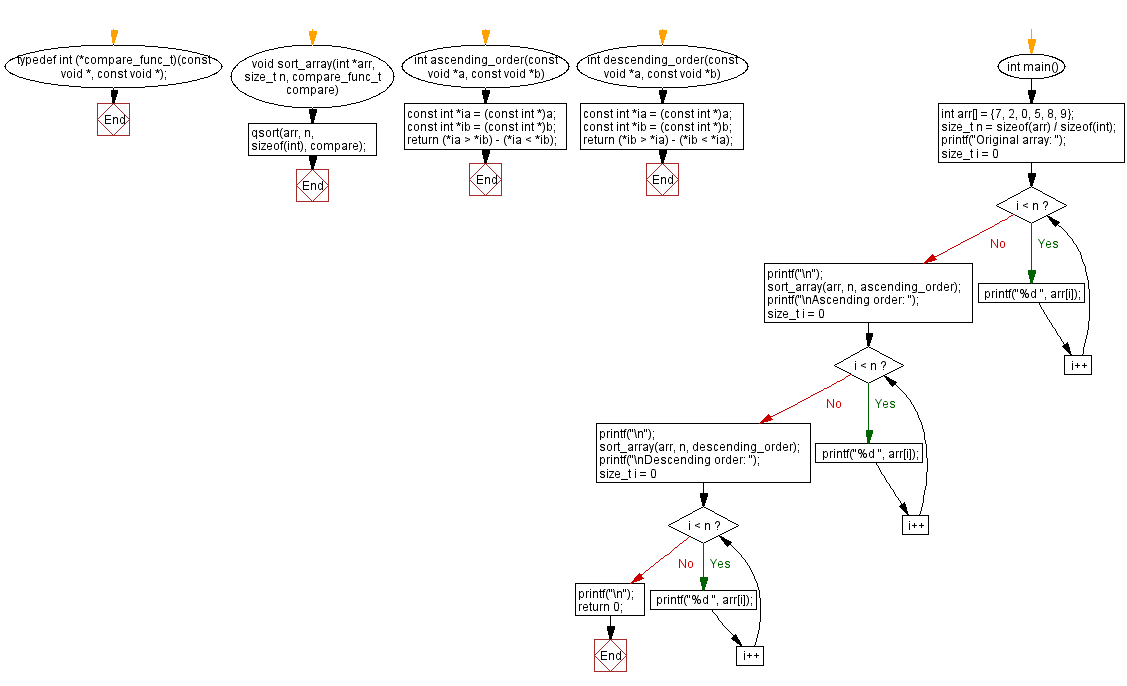
For more Practice: Solve these Related Problems:
- Write a C program to sort an array of integers based on the absolute values using a callback comparator.
- Write a C program to sort an array such that even numbers come first followed by odd numbers, using a callback function.
- Write a C program to sort an array by the number of digits in each integer using a callback function.
- Write a C program to sort an array in ascending order and then reverse it to descending order using two callbacks.
C Programming Code Editor:
Previous: Square of array elements.
Next: Sum or product of an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.