C Exercises: Square of array elements
1. Array Square Callback Variants
Write a C program to print the square of array elements using callback function.
Sample Solution:
C Code:
#include <stdio.h>
void process(int arr[], int size, int (*callback)(int))
{
for (int i = 0; i < size; i++)
{
arr[i] = callback(arr[i]);
}
}
int square(int n)
{
return n * n;
}
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
int size = sizeof(arr) / sizeof(arr[0]);
printf("Array elements before processing: ");
for (int i = 0; i < size; i++)
{
printf("%d ", arr[i]);
}
printf("\n");
process(arr, size, square);
printf("Square of the array elements after processing: ");
for (int i = 0; i < size; i++)
{
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
Sample Output:
Array elements before processing: 1 2 3 4 5 6 Square of the array elements after processing: 1 4 9 16 25 36
Explanation:
In the above example, we define a function called 'process' that takes an array of integers, a size, and a function pointer to a callback function as arguments. The 'process' function iterates over each element in the array and applies the callback function to it. The 'square' function is an example of a callback function that takes an integer as input and returns its square. In main function, we define an array of integers, call process with the array and the 'square' function as arguments, and print the results before and after processing.
In this example, the square function is a simple example of a callback function, but in practice, callback functions can be much more complex and can perform a wide range of tasks.
Flowchart:
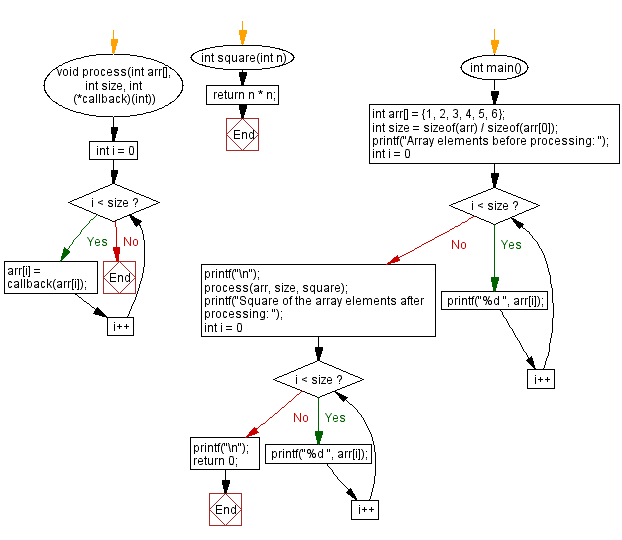
For more Practice: Solve these Related Problems:
- Write a C program to compute and print the cube of each array element using a callback function.
- Write a C program to apply a callback function that squares each element and then sums the squares.
- Write a C program to update an array in-place by replacing each element with its square using a callback.
- Write a C program to square only the prime numbers in an array using a callback function.
C Programming Code Editor:
Previous: C Callback function Exercises Home
Next: Sort an array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.