C Exercises: String is a palindrome or not
C Callback function: Exercise-4 with Solution
Write a C program to check if a string (case-sensitive) is a palindrome or not using a callback function.
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#include <ctype.h>
// Define a function pointer type that takes two characters and returns an integer.
typedef int( * compare_func_t)(char, char);
// This function checks if a given string is a palindrome.
// It takes a string, its length, and a function pointer as arguments.
int is_palindrome(char * str, size_t len, compare_func_t compare) {
// Loop through the first half of the string.
for (size_t i = 0; i < len / 2; i++) {
// Compare the i-th character from the start and end of the string using the provided comparison function.
// If they are not equal, the string is not a palindrome.
if (compare(str[i], str[len - i - 1]) != 0) {
return 0;
}
}
// If we make it through the loop without finding any non-matching characters, the string is a palindrome.
return 1;
}
// This function compares two characters in a case-sensitive manner.
// It returns -1 if the first character is less than the second, 0 if they are equal, and 1 if the first character is greater than the second.
int compare_chars(char a, char b) {
if (a < b) {
return -1;
} else if (a > b) {
return 1;
} else {
return 0;
}
}
// This function compares two characters in a case-insensitive manner.
// It returns -1 if the first character is less than the second, 0 if they are equal, and 1 if the first character is greater than the second.
// The function uses the tolower() function to convert the characters to lowercase before comparing them.
int compare_case_insensitive(char a, char b) {
a = tolower(a);
b = tolower(b);
if (a < b) {
return -1;
} else if (a > b) {
return 1;
} else {
return 0;
}
}
int main() {
char str1[] = "Madam";
size_t len = strlen(str1);
printf("String: %s", str1);
// Test the string for palindrome-ness using the case-sensitive comparison function.
if (is_palindrome(str1, len, compare_chars)) {
printf("%s is a palindrome (case-sensitive).\n", str1);
} else {
printf("\n%s is not a palindrome (case-sensitive).\n", str1);
}
// Test the string for palindrome-ness using the case-insensitive comparison function.
if (is_palindrome(str1, len, compare_case_insensitive)) {
printf("%s is a palindrome (case-insensitive).\n", str1);
} else {
printf("%s is not a palindrome (case-insensitive).\n", str1);
}
char str2[] = "aba";
len = strlen(str2);
printf("\nString: %s", str2);
// Test the string for palindrome-ness using the case-sensitive comparison function.
if (is_palindrome(str2, len, compare_chars)) {
printf("\n%s is a palindrome (case-sensitive).\n", str2);
} else {
printf("\n%s is not a palindrome (case-nsensitive).\n", str2);
}
// Test the string for palindrome-ness using the case-insensitive comparison function.
if (is_palindrome(str2, len, compare_case_insensitive)) {
printf("%s is a palindrome (case-insensitive).\n", str2);
} else {
printf("%s is not a palindrome (case-insensitive).\n", str2);
}
return 0;
}
Sample Output:
String: Madam Madam is not a palindrome (case-sensitive). Madam is a palindrome (case-insensitive). String: aba aba is a palindrome (case-sensitive). aba is a palindrome (case-insensitive).
Explanation:
The above program defines three functions: is_palindrome(), compare_chars(), and compare_case_insensitive(). It also defines a function pointer type called compare_func_t.
is_palindrome() takes a string, its length, and a function pointer as arguments. It checks if the string is a palindrome by comparing its characters using the provided comparison function. The function returns 1 if the string is a palindrome, and 0 otherwise.
compare_chars() and compare_case_insensitive() are comparison functions that take two characters as arguments and return an integer (-1, 0, or 1) based on their relative values.
Flowchart:
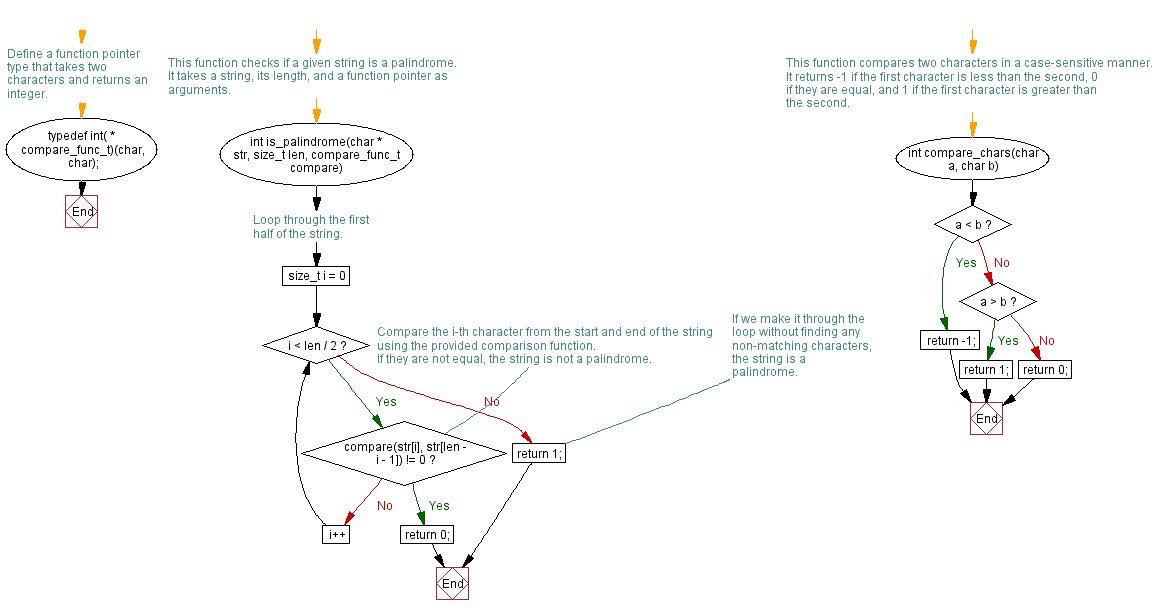
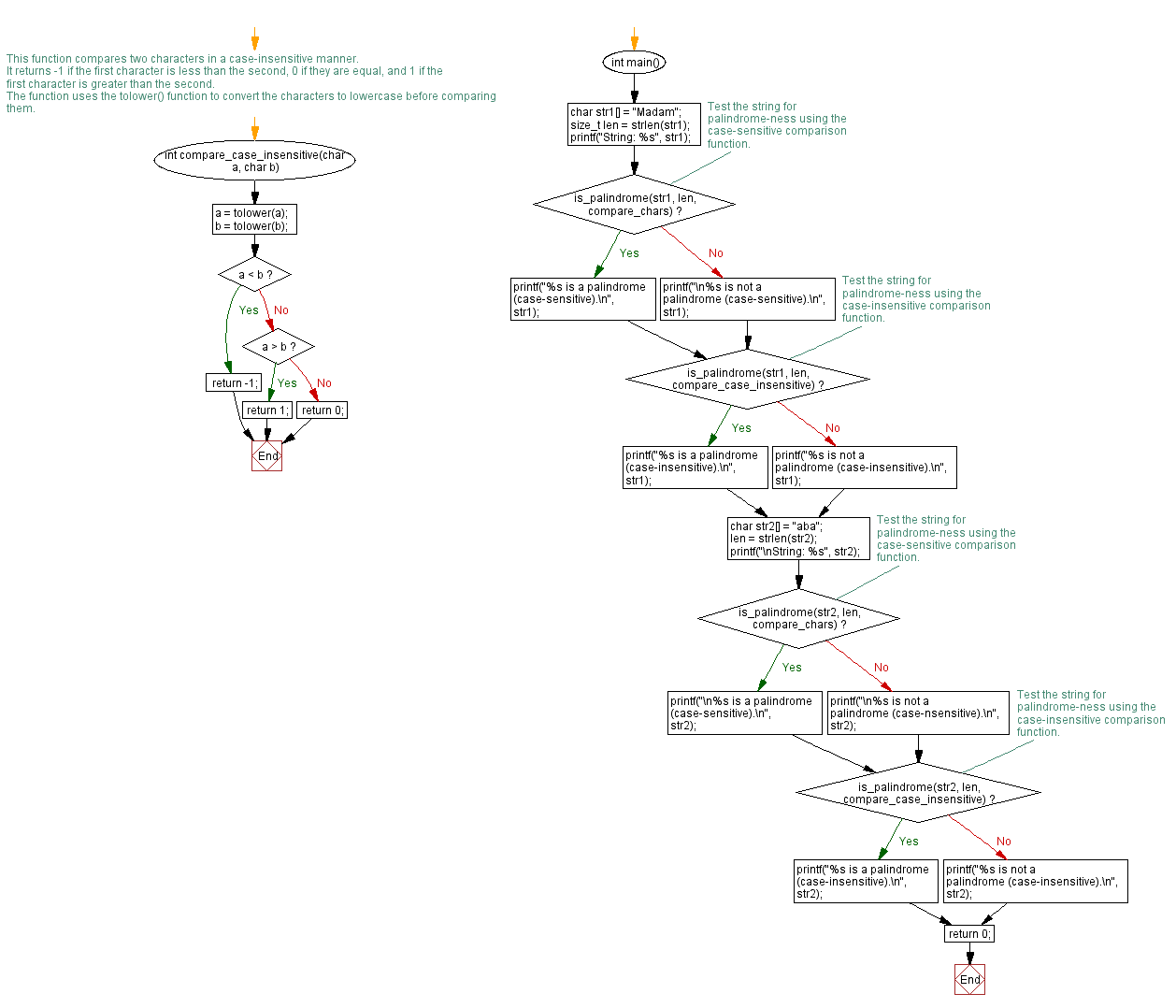
C Programming Code Editor:
Previous: Sum or product of an array of integers.
Next: Convert a string to uppercase or lowercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/callback/c-callback-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics