C Exercises: Convert a string to uppercase or lowercase
5. String Case Conversion Callback Variants
Write a program in C program to convert a string to uppercase or lowercase using a callback function to modify each character.
Sample Solution:
C Code:
#include <stdio.h>
#include <ctype.h>
void modify_string(char * str, int( * modifier)(int)) {
while ( * str != '\0') {
* str = modifier( * str);
str++;
}
}
int main() {
char str[100];
printf("Input a string: ");
fgets(str, sizeof(str), stdin);
printf("Select an option:\n");
printf("1. Convert to uppercase\n");
printf("2. Convert to lowercase\n");
int option;
scanf("%d", & option);
switch (option) {
case 1:
modify_string(str, toupper);
printf("Uppercase string: %s", str);
break;
case 2:
modify_string(str, tolower);
printf("Lowercase string: %s", str);
break;
default:
printf("Invalid option");
break;
}
return 0;
}
Sample Output:
Input a string: w3resource Select an option: 1. Convert to uppercase 2. Convert to lowercase 1 Uppercase string: W3RESOURCE ------------------------------- Input a string: JavaScript Select an option: 1. Convert to uppercase 2. Convert to lowercase 2 Lowercase string: javascript
Explanation:
In the above program modify_string() function takes a string and a function pointer ‘modifier’ as arguments. The ‘modifier’ function is used to modify each character in the string. Then loop through the string and apply the modifier function to each character using pointer arithmetic.
In the main() function, first take a string input from the user using fgets() and then ask the user to select an option to either convert the string to uppercase or lowercase. Depending on the user's choice, we call the modify_string() function with the appropriate modifier function (toupper() or tolower()). Finally, printf() function print the modified string to the console.
Flowchart:
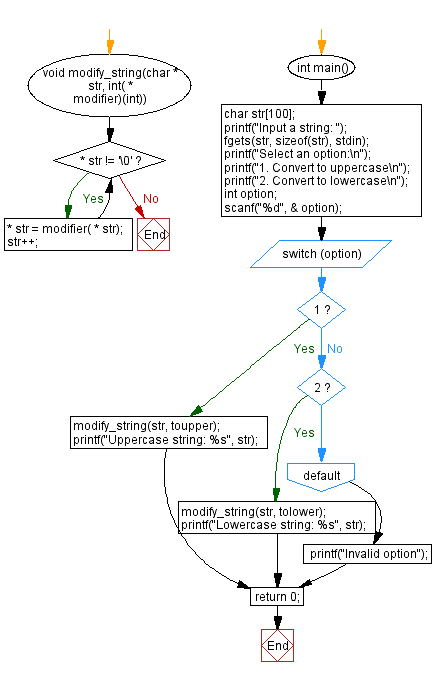
For more Practice: Solve these Related Problems:
- Write a C program to convert a string to alternating uppercase and lowercase letters using a callback function.
- Write a C program to convert only the vowels of a string to uppercase using a callback function.
- Write a C program to transform each character in a string to the next letter in the alphabet using a callback function.
- Write a C program to convert a string to lowercase and then reverse it by chaining two callback functions.
C Programming Code Editor:
Previous: String is a palindrome or not.
Next: Calculate the average or median.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.