C Exercises: Calculate the average or median
6. Average/Median Calculation Callback Variants
Write a program in C program to calculate the average or median of an array of integers using a callback function to perform the calculation.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to calculate the average of an array
double calculate_average(int arr[], int n) {
int sum = 0;
for (int i = 0; i < n; i++) {
sum += arr[i];
}
return (double) sum / n;
}
// Comparison function for sorting the array
int compare_ints(const void * a,
const void * b) {
int int_a = * ((int * ) a);
int int_b = * ((int * ) b);
return int_a - int_b;
}
// Function to calculate the median of an array
double calculate_median(int arr[], int n) {
// Sort the array in ascending order
qsort(arr, n, sizeof(int), compare_ints);
// Calculate the median
double median;
if (n % 2 == 0) {
median = (double)(arr[n / 2] + arr[n / 2 - 1]) / 2;
} else {
median = arr[n / 2];
}
return median;
}
// Function to calculate the average or median of an array
double calculate(int arr[], int n, double( * operation)(int arr[], int n)) {
return operation(arr, n);
}
int main() {
int arr[11] = {
2,
5,
4,
7,
1,
8,
4,
6,
5,
9,
10
};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original array elements: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
printf("\nSelect an option:\n");
printf("1. Calculate average of the said array elements:\n");
printf("2. Calculate median of the said array elements:\n");
int option;
scanf("%d", & option);
double result;
switch (option) {
case 1:
result = calculate(arr, n, calculate_average);
printf("Average: %f", result);
break;
case 2:
result = calculate(arr, n, calculate_median);
printf("Median: %f", result);
break;
default:
printf("Invalid option");
break;
}
return 0;
}
Sample Output:
Original array elements: 2 5 4 7 1 8 4 6 5 9 10 Select an option: 1. Calculate average of the said array elements: 2. Calculate median of the said array elements: 1 Average: 5.545455 -------------------------------- Original array elements: 2 5 4 7 1 8 4 6 5 9 10 Select an option: 1. Calculate average of the said array elements: 2. Calculate median of the said array elements: 2 Median: 5.000000
Explanation:
In the above program, we declare two functions to perform the calculations: a) calculate_average() function to calculate the average of an array. b) calculate_median() function to calculate the median of an array. We also define a comparison function compare_ints() that is used by qsort() to sort the array in ascending order.
We then define a function calculate() that takes three arguments the array, the number of elements in the array, and a callback function ‘operation’. This function calls the ‘operation’ function to perform the calculation on the array.
In the main() function, we first define an array of integers and the number of elements in the array. Then, we ask the user to select an option to either calculate the average or median of the array. Depending on the user's choice, we call the calculate() function with the appropriate callback function (calculate_average() or calculate_median()). Finally, printf() function print the result to the console.
Flowchart:
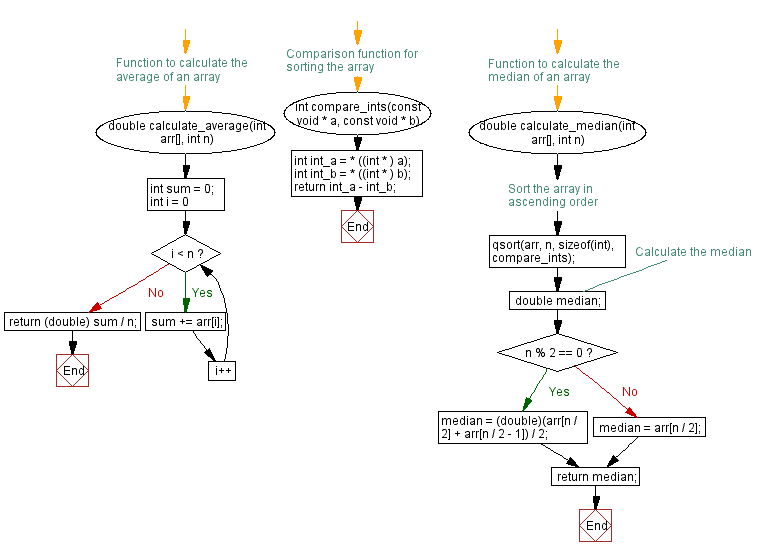
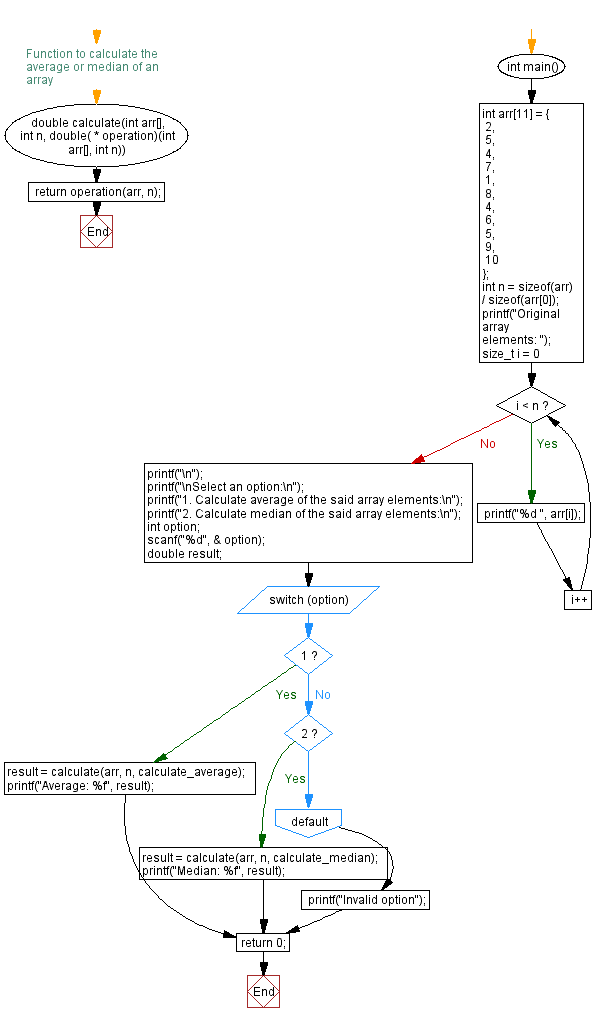
For more Practice: Solve these Related Problems:
- Write a C program to calculate the mode of an array using a callback function that tallies frequency.
- Write a C program to compute the standard deviation of an array by applying a callback function for summing squared differences.
- Write a C program to determine both the average and the range of an array using a callback function.
- Write a C program to compute a weighted average of array elements using a callback function that applies custom weights.
C Programming Code Editor:
Previous: Convert a string to uppercase or lowercase.
Next: Remove all whitespace from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.