C Exercises: Find the prime numbers within a range of numbers
34. Prime Numbers within a Range
Write a program in C to find the prime numbers within a range of numbers.
The program should prompt the user to input the lower and upper bounds of the range. It will then check each number in this range to determine if it is prime, and display all the prime numbers found within these limits.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main(){
int num,i,ctr,stno,enno; // Declare variables for the number, loop counters, and range.
printf("Input starting number of range: "); // Prompt the user to input the starting number of the range.
scanf("%d",&stno); // Read the input from the user.
printf("Input ending number of range : "); // Prompt the user to input the ending number of the range.
scanf("%d",&enno); // Read the input from the user.
printf("The prime numbers between %d and %d are : \n",stno,enno); // Print the message indicating the range.
for(num = stno; num <= enno; num++) // Loop through the numbers in the specified range.
{
ctr = 0; // Initialize the counter.
for(i = 2; i <= num/2; i++) // Loop through possible divisors.
{
if(num % i == 0) // If a divisor is found...
{
ctr++; // ...increment the counter.
break; // Exit the loop.
}
}
if(ctr == 0 && num != 1) // If no divisors were found and the number is not 1...
printf("%d ",num); // ...print the prime number.
}
printf("\n"); // Move to the next line after printing all prime numbers.
}
Output:
Input starting number of range: 1 Input ending number of range : 50 The prime numbers between 1 and 50 are : 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
Flowchart:
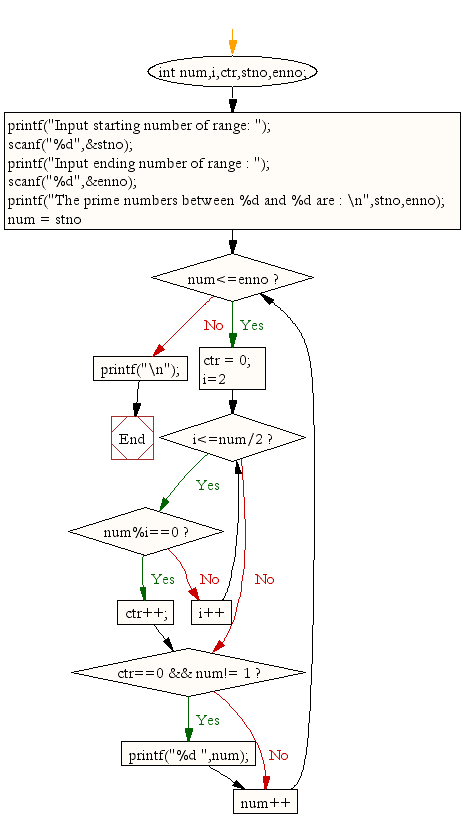
For more Practice: Solve these Related Problems:
- Write a C program to find all prime numbers in a given range using the Sieve of Eratosthenes.
- Write a C program to list prime numbers within a range and display their total count.
- Write a C program to compute the sum of all prime numbers within a specified range.
- Write a C program to find primes in a range using recursive prime-checking.
C Programming Code Editor:
Previous: Write a C program to display Pascal's triangle
Next: Write a program in C to display the first n terms of Fibonacci series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.