C Exercises: Display Pascal's triangle
33. Pascal's Triangle Display
Write a C program to display Pascal's triangle.
From Wikipedia,
In mathematics, Pascal's triangle is a triangular array of the binomial coefficients which play a crucial role in probability theory, combinatorics, and algebra. In much of the Western world, it is named after the French mathematician Blaise Pascal, although other mathematicians studied it centuries before him in Persia, India, China, Germany, and Italy.
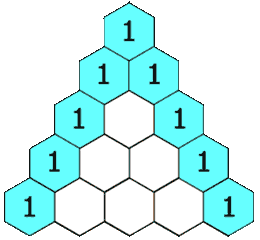
Source: Wikipedia
Properties and Applications:
Binomial coefficients:
Each number in Pascal's Triangle represents a binomial coefficient. For example, the third row corresponds to the coefficients of the expansion of (a+b)2, which are 1, 2, 1.
Combinations:
The numbers in Pascal's Triangle can be used to find combinations. The number in the 𝑛n-th row and 𝑘-th column (0-indexed) is , the number of ways to choose 𝑘 elements from a set of 𝑛 elements.
Symmetry:
Pascal's Triangle is symmetric. The left half is a mirror image of the right half.
Sum of Rows:
The sum of the elements in the 𝑛-th row is 2𝑛.
Fibonacci Sequence:
Diagonal sums of Pascal's Triangle generate the Fibonacci sequence.
Pascal's Triangle has many fascinating properties and connections to various areas of mathematics, making it a fundamental and widely studied concept.
Construction of Pascal's Triangle:
As shown in Pascal's triangle, each element is equal to the sum of the two numbers immediately above it.
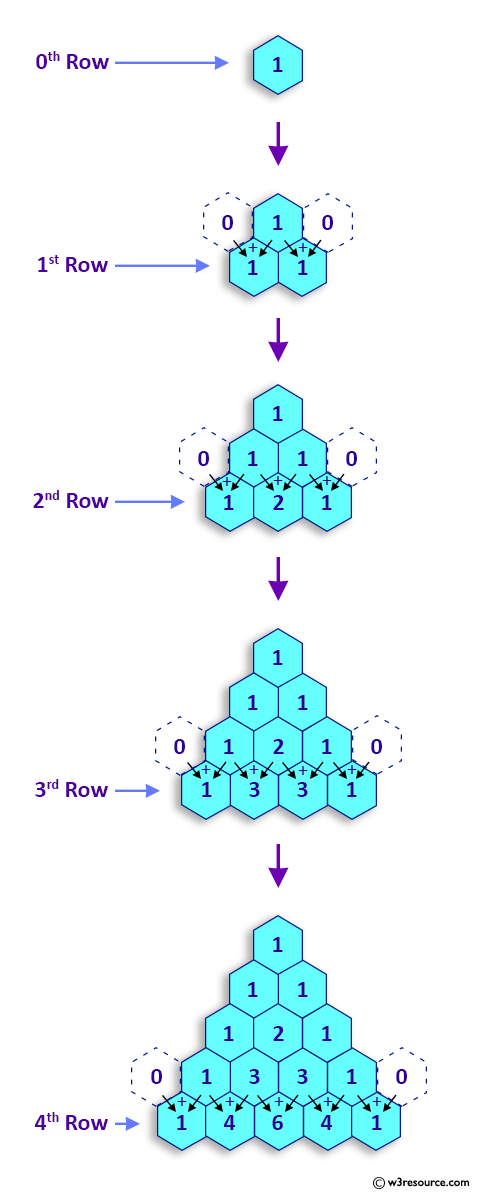
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int no_row,c=1,blk,i,j; // Declare variables for row count, pattern counter, and loop control.
printf("Input number of rows: "); // Prompt the user to input the number of rows.
scanf("%d",&no_row); // Read the input from the user.
for(i=0;i<no_row;i++) // Outer loop for iterating over rows.
{
for(blk=1;blk<=no_row-i;blk++) // Inner loop for printing spaces.
printf(" ");
for(j=0;j<=i;j++) // Inner loop for generating and printing pattern.
{
if (j==0||i==0) // If it's the first column or first row, set c to 1.
c=1;
else
c=c*(i-j+1)/j; // Calculate the next pattern value.
printf("% 4d",c); // Print the pattern value.
}
printf("\n"); // Move to the next row.
}
}
Output:
Input number of rows: 5 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Explanation:
- Include Library:
- #include <stdio.h>: Includes the standard input/output library.
- Main Function Declaration:
- void main(): Declares the main function of the program.
- Variable Declaration:
- int no_row, c=1, blk, i, j;: Declares integer variables for the number of rows (no_row), the coefficient ('c'), block spacing (blk), and loop counters ('i' and 'j').
- User Input:
- printf("Input number of rows: ");: Prompts the user to input the number of rows.
- scanf("%d", &no_row);: Reads the user input and stores it in 'no_row'.
- Outer Loop (Rows):
- for(i=0; i<no_row; i++): Loops from 0 to 'no_row - 1' to iterate over each row.
- Inner Loop 1 (Spaces):
- for(blk=1; blk<=no_row-i; blk++): Loops to print leading spaces for alignment.
- printf(" ");: Prints two spaces for each block.
- Inner Loop 2 (Pascal's Triangle Values):
- for(j=0; j<=i; j++): Loops to calculate and print values in the current row of Pascal's triangle.
- if (j==0 || i==0) c=1;: Sets 'c' to 1 for the first element in the row.
- else c=c*(i-j+1)/j;: Calculates the Pascal's triangle value using the formula for binomial coefficients.
- printf("% 4d", c);: Prints the value of 'c' with a width of 4 characters for proper alignment.
- New Line:
- printf("\n");: Moves to the next line after printing all values in the current row.
- End of Program:
- The program terminates after printing the Pascal's triangle up to the specified number of rows.
Flowchart:
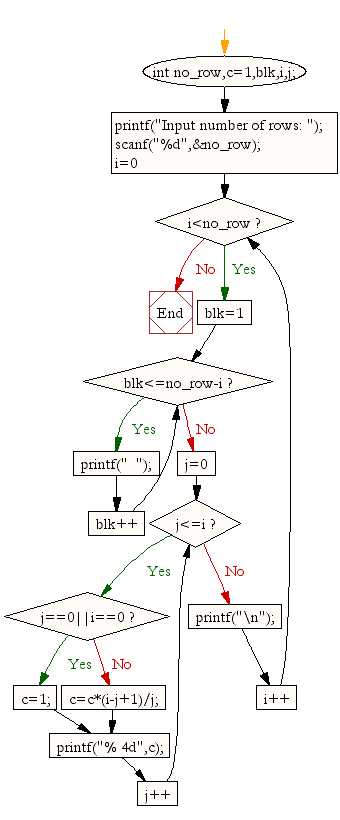
Frequently Asked Questions on Pascal's Triangle
1. What is Pascal's Triangle?
Pascal's Triangle is a triangular array of numbers where each number is the sum of the two numbers directly above it. It is named after the French mathematician Blaise Pascal, though it was studied by many mathematicians before him.
2. How is Pascal's Triangle constructed?
Start with a single "1" at the top. Each subsequent row starts and ends with "1". Every other number in the triangle is the sum of the two numbers directly above it from the previous row.
3. What are some properties of Pascal's Triangle?
Each row represents the coefficients of the binomial expansion.
The triangle is symmetric.
The sum of the elements of the n-th row is 2^n.
The elements of the n-th row are binomial coefficients .
4. How can Pascal's Triangle be used in binomial expansions?
The n-th row of Pascal's Triangle gives the coefficients for the expansion of (a + b)^n. For example, the 3rd row "1 3 3 1" corresponds to (a + b)^3 = 1a^3 + 3a^2b + 3ab^2 + 1b^3 .
5. What are binomial coefficients?
Binomial coefficients, denoted as , are the coefficients of the terms in the expansion of (a + b)^n. They can be found in Pascal's Triangle and calculated using the formula
6. Can Pascal's Triangle be used to find combinations?
Yes, the values in Pascal's Triangle correspond to combinations. The value at row n and position k (starting from 0) is , which represents the number of ways to choose k elements from a set of n elements.
7. How do the Fibonacci sequence and Pascal's Triangle relate?
The Fibonacci sequence can be found by summing the shallow diagonals of Pascal's Triangle. For example, the sequence 1, 1, 2, 3, 5, 8,... can be obtained by adding 1, 1, (1+1), (1+2), (1+3), (1+3+2),...
8. What are some applications of Pascal's Triangle?
Pascal's Triangle is used in algebra (binomial expansions), probability theory (combinations), and even in computing (dynamic programming).
9. How is Pascal's Triangle related to Sierpinski's Triangle?
If you color the odd numbers in Pascal's Triangle, the pattern that emerges is known as Sierpinski's Triangle, a famous fractal pattern.
10. How do you find the row sum in Pascal's Triangle?
The sum of the elements in the n-th row of Pascal's Triangle is 2^n.
11. Can Pascal's Triangle be extended to negative or non-integer rows?
Traditionally, Pascal's Triangle is defined for non-negative integers. However, extensions to negative or non-integer rows involve more advanced mathematical concepts and are less commonly used.
12. How can I generate Pascal's Triangle programmatically?
Pascal's Triangle can be generated using simple iterative methods or recursion in programming languages like Python, C, and Java. It involves calculating binomial coefficients or using previously computed rows to generate new ones.
13. Is there a closed-form formula for elements in Pascal's Triangle?
Yes, the element at row n and position k is given by the binomial coefficient
14. What are the diagonal properties of Pascal's Triangle?
The diagonals of Pascal's Triangle correspond to figurate numbers: the first diagonal is all 1s, the second diagonal is counting numbers, the third diagonal is triangular numbers, and so forth.
For more Practice: Solve these Related Problems:
- Write a C program to display Pascal's triangle using recursion to calculate binomial coefficients.
- Write a C program to print Pascal's triangle and compute the sum of the numbers in each row.
- Write a C program to display Pascal's triangle using iterative loops with proper formatting.
- Write a C program to generate Pascal's triangle and then print it in reverse order.
C Programming Code Editor:
Previous: Write a C program to determine whether a given number is prime or not.
Next: Write a program in C to find the prime numbers within a range of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.