C Exercises: Counts the number of characters left in the file
C For Loop: Exercise-60 with Solution
Write a C program that takes user input and counts the number of characters until the end of the file.
Note: On Linux systems and OS X, the character to input to cause an EOF is CTRL+D. For Windows, it's CTRL+Z
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
int main(void)
{
// Variable declaration and initialization
int ctr = 0;
// Prompting the user for input
printf("\nInput characters: On Linux systems and OS X EOF is CTRL+D. For Windows EOF is CTRL+Z.\n");
// Loop to count characters until EOF (End of File) is encountered
while (getchar() != EOF)
{
ctr++;
}
// Printing the number of characters counted
printf("\nNumber of Characters: %d\n", ctr);
return 0;
}
Sample Output:
Input characters: On Linux systems and OS X EOF is CTRL+D. For Windows EOF is CTRL+Z. Number of Characters: 10
Flowchart:
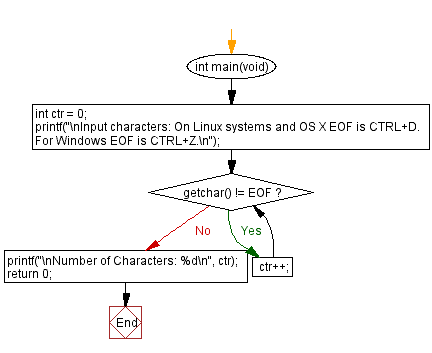
C Programming Code Editor:
Previous: Check whether an n digits number is Armstrong or not.
Next: Count the upper-case, lower-case and other characters
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics