C Exercises: Check whether an n digits number is Armstrong or not
59. Armstrong Number Check for n Digits
Write a C program to check the Armstrong number of n digits.
This C program checks whether a given n-digit number is an Armstrong number or not. It prompts the user for an integer input, calculates the number of digits in the input number, and then computes the sum of cubes of individual digits raised to the power of 'n'. Finally, it compares this result with the original number to determine whether it's an Armstrong number or not, printing the result accordingly.
Visual Presentation:
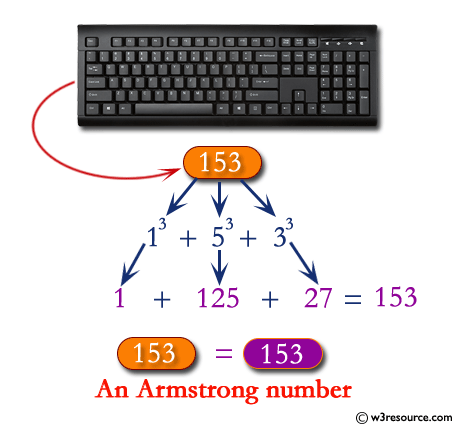
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <math.h> // Include the math header file.
int main()
{
// Variable declarations
int n1, onum, r, result = 0, n = 0 ;
// Prompting user for input
printf("\n\n Check whether an n digits number is armstrong or not :\n");
printf("-----------------------------------------------------------\n");
printf(" Input an integer : ");
scanf("%d", &n1);
// Store the original number for later comparison
onum = n1;
// Count the number of digits in the input number
while (onum != 0)
{
onum /= 10;
++n;
}
// Reset onum to the original number for further processing
onum = n1;
// Calculate the sum of cubes of individual digits raised to the power of 'n'
while (onum != 0)
{
r = onum % 10;
result += pow(r, n);
onum /= 10;
}
// Check if the result is equal to the original number
if(result == n1)
printf(" %d is an Armstrong number.\n\n", n1);
else
printf(" %d is not an Armstrong number.\n\n", n1);
return 0;
}
Output:
Check whether an n digits number is Armstrong or not : ----------------------------------------------------------- Input an integer : 1634 1634 is an Armstrong number.
Flowchart:
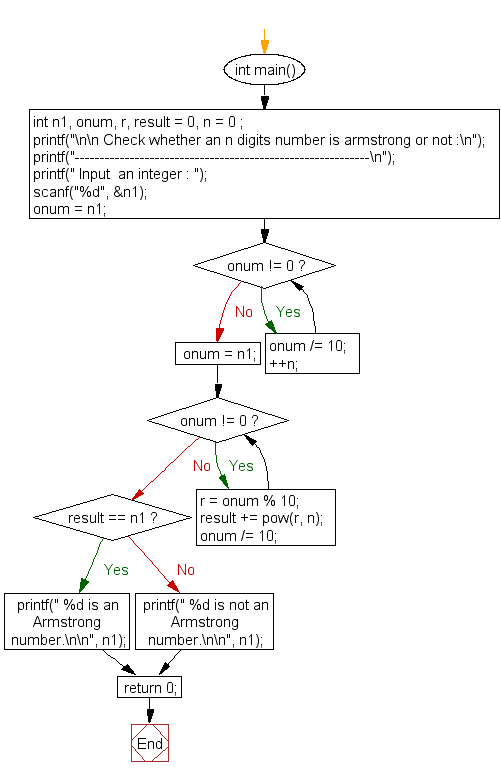
For more Practice: Solve these Related Problems:
- Write a C program to check if a number with n digits is an Armstrong number using recursion.
- Write a C program to verify if a number is Armstrong by dynamically computing the power based on digit count.
- Write a C program to check if a number is Armstrong and then display the sum of its digits raised to the power of n.
- Write a C program to determine if a number is Armstrong without converting it to a string.
C Programming Code Editor:
Previous: Find the length of a string without using the library function.
Next: Counts the number of characters left in the file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.