C Exercises: Count the upper-case, lower-case and other characters
61. Count Uppercase, Lowercase, and Other Characters
Write a C program that takes input from the user and counts the number of uppercase and lowercase letters, as well as the number of other characters.
Note: To exit On Linux systems and OS X, the character to input to cause an EOF is CTRL+D. For Windows, it's CTRL+Z
This C program prompts the user to input a string. It then iterates through each character of the input string, counting the number of uppercase letters, lowercase letters, and other characters (such as digits, symbols, or whitespace). Finally, it prints out the counts of uppercase letters, lowercase letters, and other characters.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <ctype.h>
int main(void)
{
// Variable declarations and initialization
int chr;
int uppercase_ctr = 0, lowercase_ctr = 0, other_ctr = 0;
// Prompting the user for input
printf("\nInput characters: On Linux systems and OS X EOF is CTRL+D. For Windows EOF is CTRL+Z.\n");
// Loop to process characters until EOF (End of File) is encountered
while ((chr = getchar()) != EOF)
{
// Checking if the character is uppercase
if (isupper(chr))
uppercase_ctr++;
// Checking if the character is lowercase
else if (islower(chr))
lowercase_ctr++;
// If it's not uppercase or lowercase, consider it as another character
else
other_ctr++;
}
// Printing the counts of different types of characters
printf("\nUppercase letters: %d\n", uppercase_ctr);
printf("Lowercase letters: %d\n", lowercase_ctr);
printf("Other characters: %d\n", other_ctr);
return 0;
}
Output:
Input characters: On Linux systems and OS X EOF is CTRL+D. For Windows EOF is CTRL+Z. Uppercase letters: 0 Lowercase letters: 9 Other characters: 1
Flowchart:
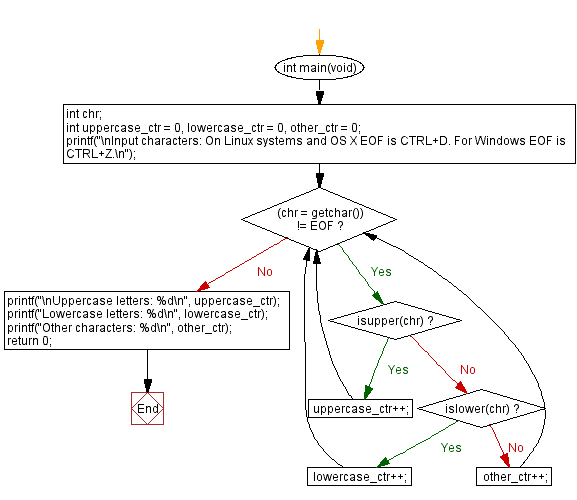
For more Practice: Solve these Related Problems:
- Write a C program to count the number of uppercase, lowercase, digits, and special characters in user input.
- Write a C program to read input until EOF and count uppercase and lowercase letters, then display their percentages.
- Write a C program to count characters by type and output a simple bar chart representing the counts.
- Write a C program to count the number of uppercase, lowercase, and other characters, then sort the counts in descending order.
C Programming Code Editor:
Previous: Counts the number of characters left in the file.
Next: C Searching and Sorting Algorithm Exercises Home
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.