C Exercises: Find the number of trailing zeroes in a given factorial
10. Trailing Zeros in Factorial Variants
Write a C program to find the number of trailing zeroes in a given factorial.
Example 1:
Input: 4
Output: 0
Explanation: 4! = 24, no trailing zero.
Example 2:
Input: 6
Output: 1
Explanation: 6! = 720, one trailing zero.
Example:
Input:
n = 4
n = 5
Output:
Number of trailing zeroes of factorial 4 is 0
Number of trailing zeroes of factorial 5 is 1
Visual Presentation:
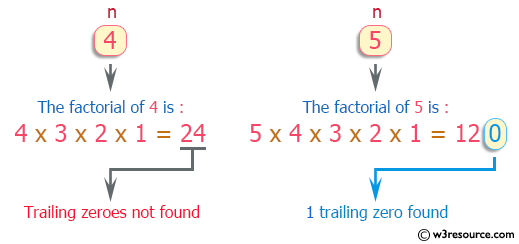
Sample Solution:
C Code:
#include <stdio.h>
// Function to count the number of trailing zeroes in the factorial of a number
static int trailing_Zeroes(int n) {
int number = 0;
// Loop to calculate the number of trailing zeroes
while (n > 0) {
number += n / 5; // Count how many multiples of 5 are present in the factorial
n /= 5; // Divide the number by 5 to check for the next multiple of 5
}
return number; // Return the count of trailing zeroes
}
// Main function to test the trailing_Zeroes function with different values
int main(void) {
int n = 4;
printf("\nNumber of trailing zeroes of factorial %d is %d ", n, trailing_Zeroes(n)); // Display the number of trailing zeroes for n
n = 5;
printf("\nNumber of trailing zeroes of factorial %d is %d ", n, trailing_Zeroes(n)); // Display the number of trailing zeroes for n
return 0;
}
Sample Output:
Number of trailing zeroes of factorial 4 is 0 Number of trailing zeroes of factorial 5 is 1
Flowchart:
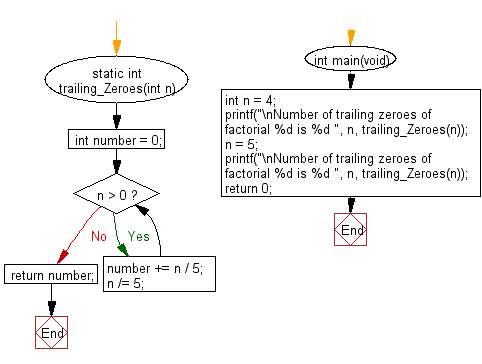
For more Practice: Solve these Related Problems:
- Write a C program to calculate the number of trailing zeros in n! by counting factors of 5.
- Write a C program to compute trailing zeros in a factorial using iterative division by powers of 5.
- Write a C program to find trailing zeros in large factorials by optimizing the factor counting process.
- Write a C program to display both the factorial value (if small) and its trailing zero count for comparison.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to get the column number (integer value) that corresponds to a column title as appear in an Excel sheet.
Next: Write a C program to count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.