C Exercises: Get the column number that corresponds to a column title as appear in an Excel sheet
C Programming Mathematics: Exercise-9 with Solution
Write a C program to get the column number (integer value) that corresponds to a column title as it appears in an Excel sheet.
For example:
A -> 1
B -> 2
C -> 3
...
Z -> 26
AA -> 27
AB -> 28
...
Example:
Input:
col_title1[ ] ="C"
col_title2[ ] ="AC"
col_title3[ ] ="ZY"
Output:
Corresponding number: 3
Corresponding number: 29
Corresponding number: 701
Visual Presentation:
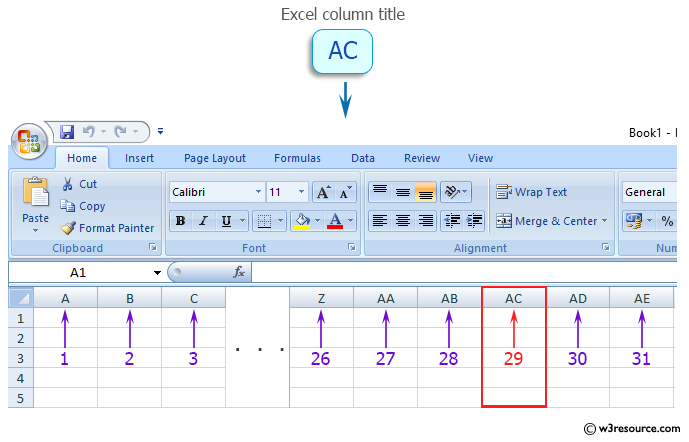
Sample Solution:
C Code:
#include <stdio.h>
// Function to convert an Excel column title to a corresponding number
int excel_title_to_Number(char* s) {
int k = 0;
// Loop to convert Excel column title to a number
while (s && *s) {
k = k * 26 + (*s) - 'A' + 1; // Convert each character to its corresponding value in Excel column naming scheme
s++; // Move to the next character in the title
}
return k; // Return the corresponding number
}
// Main function to test the excel_title_to_Number function with different titles
int main(void) {
char col_title1[] = "C";
printf("\nExcel column title: = %s", col_title1);
printf("\nCorresponding number: %d ", excel_title_to_Number(col_title1)); // Display the corresponding number for the title
char col_title2[] = "AC";
printf("\n\nExcel column title: = %s", col_title2);
printf("\nCorresponding number: %d ", excel_title_to_Number(col_title2)); // Display the corresponding number for the title
char col_title3[] = "ZY";
printf("\n\nExcel column title: = %s", col_title3);
printf("\nCorresponding number: %d ", excel_title_to_Number(col_title3)); // Display the corresponding number for the title
return 0;
}
Sample Output:
Excel column title: = C Corresponding number: 3 Excel column title: = AC Corresponding number: 29 Excel column title: = ZY Corresponding number: 701
Flowchart:
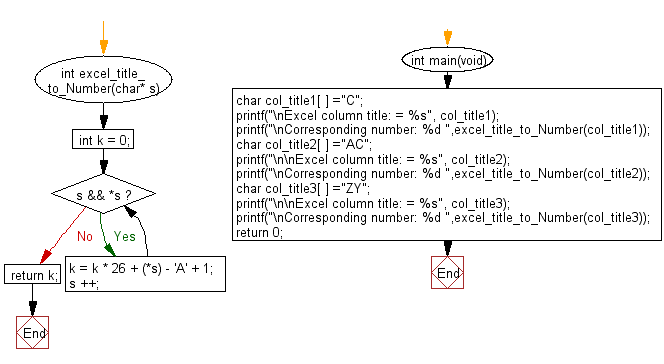
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to get the Excel column title that corresponds to a given column number.
Next: Write a C program to find the number of trailing zeroes in a given factorial.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics