C Exercises: Get the Excel column title that corresponds to a given column number
C Programming Mathematics: Exercise-8 with Solution
Write a C program to get the Excel column title that corresponds to a given column number (integer value).
For example:
1 -> A
2 -> B
3 -> C
...
26 -> Z
27 -> AA
28 -> AB
...
Example:
Input:
n = 3
n = 27
n = 151
Output:
Excel column title: C
Excel column title: AA
Excel column title: EU
Visual Presentation:
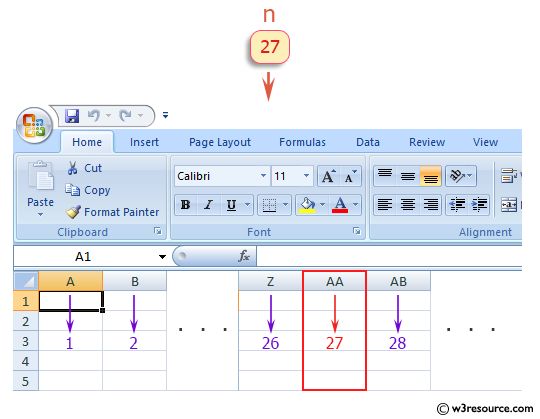
Sample Solution:
C Code:
#include <stdio.h>
// Function to convert a column number to an Excel column title
static char *convert_To_Excel_Title(int column_no) {
if (column_no <= 0) {
return ""; // Return an empty string for invalid column numbers
}
char *result = malloc(1024); // Allocate memory to store the Excel column title
int len = 0;
// Loop to convert column number to Excel column title
do {
result[len++] = ((column_no - 1) % 26) + 'A'; // Get the character corresponding to the column number
column_no = (column_no - 1) / 26; // Update column number for the next iteration
} while (column_no > 0);
result[len] = '\0'; // Null-terminate the resulting string
// Reverse the characters in the string to get the correct Excel column title
int i, j;
for (i = 0, j = len - 1; i < j; i++, j--) {
char c = result[i];
result[i] = result[j];
result[j] = c;
}
return result; // Return the Excel column title string
}
// Main function to test the convert_To_Excel_Title function with different values
int main(void) {
int n = 3;
printf("\nColumn Number n = %d", n);
printf("\nExcel column title: %s ", convert_To_Excel_Title(n)); // Display Excel column title for n
n = 27;
printf("\n\nColumn Number n = %d", n);
printf("\nExcel column title: %s ", convert_To_Excel_Title(n)); // Display Excel column title for n
n = 151;
printf("\n\nColumn Number n = %d", n);
printf("\nExcel column title: %s ", convert_To_Excel_Title(n)); // Display Excel column title for n
return 0;
}
Sample Output:
Column Number n = 3 Excel column title: C Column Number n = 27 Excel column title: AA Column Number n = 151 Excel column title: EU
Flowchart:
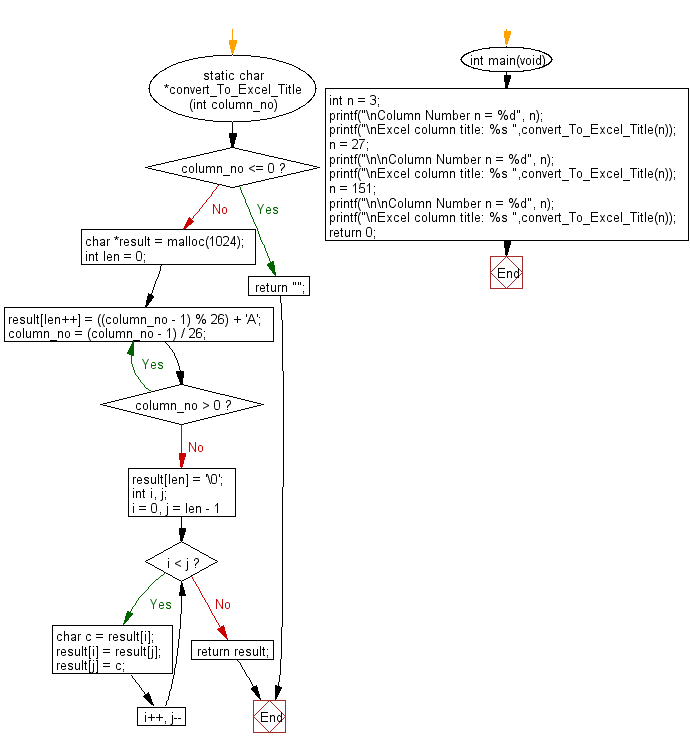
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to get the fraction part from two given integers representing the numerator and denominator in string format.
Next: Write a C program to get the column number (integer value) that corresponds to a column title as appear in an Excel sheet.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics