C Exercises: Get the fraction part from two given integers representing the numerator and denominator in string format
7. Fractional Part Extraction Variants
Write a C program to get the fraction part from two given integers representing the numerator and denominator in string format.
Example:
Input:
n = 3
d = 2
n = 4
d = 7
Output:
Fractional part: 1.5
Fractional part: 0.(571428)
Sample Solution:
C Code:
//Source: https://bit.ly/2KNsta8
// Header files inclusion
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
// Function to convert a fraction to a decimal string
char* fractionToDecimal(int numerator, int denominator) {
char *p; // Pointer for the resulting string
int psz, n, *dec, dsz, x; // Variables for string sizes and calculations
long long num, den, k, f; // Variables for numerator, denominator, and calculations
int i, repeat_at; // Loop control and repeat position variables
int neg = 0; // Flag for negative result
psz = dsz = 100; // Initial size for string buffers
n = x = 0; // Initialize counters
p = malloc(psz * sizeof(char)); // Allocate memory for the resulting string
//assert(p); // Uncommented assert - check for allocation success
// Determine if the result will be negative
neg = ((numerator > 0 && denominator < 0) ||
(numerator < 0 && denominator > 0)) ? 1 : 0;
num = numerator;
den = denominator;
num = (num < 0) ? -num : num; // Make numerator positive
den = (den < 0) ? -den : den; // Make denominator positive
// Calculate the integer part of the result
k = num / den;
f = num % den;
// If the result is negative and either the integer part or fractional part exists, append a negative sign
if (neg && (k || f)) p[n++] = '-';
// Append the integer part to the result string
n += sprintf(&p[n], "%lld", k);
if (!f) {
p[n] = 0;
return p;
}
// If fractional part exists, append a decimal point
p[n++] = '.';
// Allocate memory for an array to store fractional digits and their positions
dec = malloc(dsz * sizeof(int));
//assert(dec); // Uncommented assert - check for allocation success
repeat_at = -1; // Initialize repeat position
if (f < 0) f = -f; // Make the fractional part positive
// Loop to convert fraction to decimal
while (f) {
// Check for repeating digits in the fractional part
for (i = 0; i < x; i += 2) {
if (dec[i] == f) {
repeat_at = i;
goto done;
}
}
// If no repeating digits found, continue converting
if (x + 1 >= dsz) {
dsz *= 2;
dec = realloc(dec, dsz * sizeof(int)); // Reallocate memory for the array
//assert(dec); // Uncommented assert - check for allocation success
}
// Store current fractional digit and its position
dec[x++] = f;
f *= 10;
k = f / den;
dec[x++] = k;
f = f % den;
}
done:
// Loop to construct the decimal string
for (i = 0; i < x; i += 2) {
// Check if the string buffer needs resizing
if (n + 3 > psz) {
psz *= 2;
p = realloc(p, psz * sizeof(char)); // Reallocate memory for the string buffer
//assert(p); // Uncommented assert - check for allocation success
}
// Add digits to the string and place '(' for repeating digits
if (repeat_at == i) {
p[n++] = '(';
}
p[n++] = '0' + dec[i + 1];
}
// Add ')' if repeating digits were found
if (repeat_at != -1) p[n++] = ')';
p[n++] = 0; // Null-terminate the string
free(dec); // Free memory allocated for the fractional digits array
return p; // Return the resulting string
}
// Main function to test the fractionToDecimal function with different values
int main(void) {
int n = 3;
int d = 2;
printf("\nn = %d, d = %d ", n, d);
printf("\nFractional part: %s ", fractionToDecimal(n, d)); // Display result for n/d
n = 4;
d = 7;
printf("\n\nn = %d, d = %d ", n, d);
printf("\nFractional part: %s ", fractionToDecimal(n, d)); // Display result for n/d
return 0;
}
Sample Output:
n = 3, d = 2 Fractional part: 1.5 n = 4, d = 7 Fractional part: 0.(571428)
Flowchart:
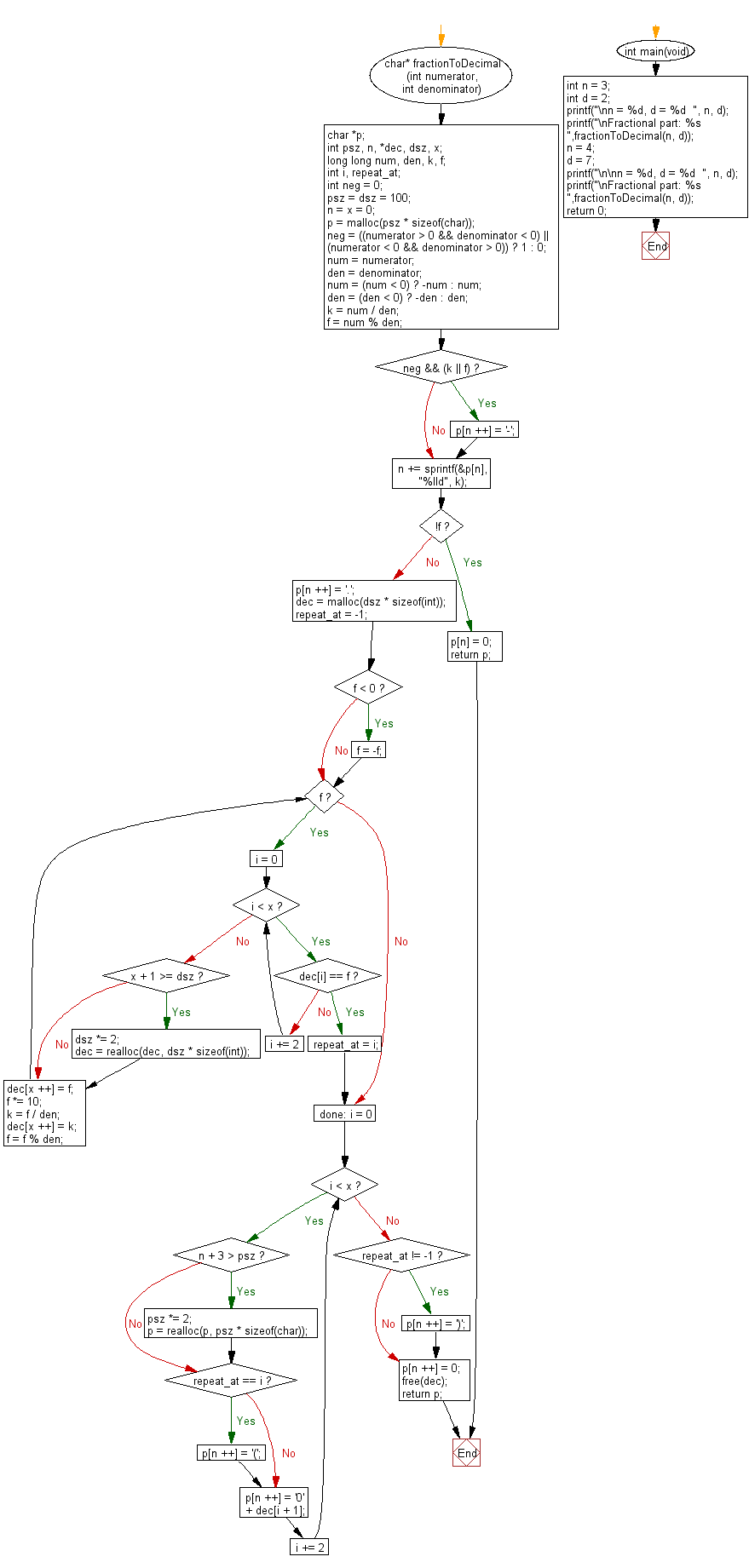
For more Practice: Solve these Related Problems:
- Write a C program to compute the fractional part of a division and represent recurring decimals with parentheses.
- Write a C program to extract the fractional part from two integers and format the result as a mixed fraction.
- Write a C program to calculate and display the fractional part by performing long division manually.
- Write a C program to convert the fraction obtained from two integers into a string showing non-repeating and repeating parts.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to check if a given string can be interpreted as a decimal number.
Next: Write a C program to get the Excel column title that corresponds to a given column number (integer value).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.