C Exercises: Check if a given string can be interpreted as a decimal number
6. Decimal Number String Check Variants
Write a C program to check if a given string can be interpreted as a decimal number.
Example:
Input:
str_num1[ ] ="1234"
str_num2[ ]=" 0.1 "
str_num3[ ]=" -90e3 "
str_num4[ ]=" 99e2.5 "
Output:
Is the above string is a number? 1
Is the above string is a number? 1
Is the above string is a number? 1
Is the above string is a number? 0
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
// Function to determine if a given string is a valid number
bool is_Number(char* str1) {
int n, m;
// Skip leading spaces
while (*str1 == ' ') str1 ++;
n = m = 0;
// Skip the sign of the number
if (*str1 == '+' || *str1 == '-') str1 ++;
// Loop to check for digits before a possible decimal point
while (*str1 >= '0' && *str1 <= '9') {
n ++;
str1 ++;
}
// Check if there is a decimal point
if (*str1 == '.') {
str1 ++;
// Loop to check for digits after the decimal point
while (*str1 >= '0' && *str1 <= '9') {
m ++;
str1 ++;
}
// If no digits found before or after the decimal point, return false
if (!n && !m) return false;
} else if (!n) {
// If no digits found before a decimal point, return false
return false;
}
// Check for exponent notation (e.g., 1.23e45)
if (*str1 == 'e' || *str1 == 'E') {
str1 ++;
// Check for sign after 'e' or 'E'
if (*str1 == '+' || *str1 == '-') str1 ++;
n = 0;
// Loop to check for digits in the exponent
while (*str1 >= '0' && *str1 <= '9') {
n ++;
str1 ++;
}
// If no digits found in the exponent, return false
if (!n) return false;
}
// Skip trailing spaces
while (*str1 == ' ') str1 ++;
// Return true if the entire string has been checked and no invalid characters found
return *str1 == 0 ? true : false;
}
// Main function to test the is_Number function with different strings
int main(void) {
char str_num1[] ="1234";
printf("\nstr_num = %s", str_num1);
printf("\nIs the above string a number? %d ", is_Number(str_num1));
char str_num2[]=" 0.1 ";
printf("\n\nstr_num = %s", str_num2);
printf("\nIs the above string a number? %d ", is_Number(str_num2));
char str_num3[]=" -90e3 ";
printf("\n\nstr_num = %s", str_num3);
printf("\nIs the above string a number? %d ", is_Number(str_num3));
char str_num4[]=" 99e2.5 ";
printf("\n\nstr_num = %s", str_num4);
printf("\nIs the above string a number? %d ", is_Number(str_num4));
return 0;
}
Sample Output:
str_num = 1234 Is the above string is a number? 1 str_num = 0.1 Is the above string is a number? 1 str_num = -90e3 Is the above string is a number? 1 str_num = 99e2.5 Is the above string is a number? 0
Flowchart:
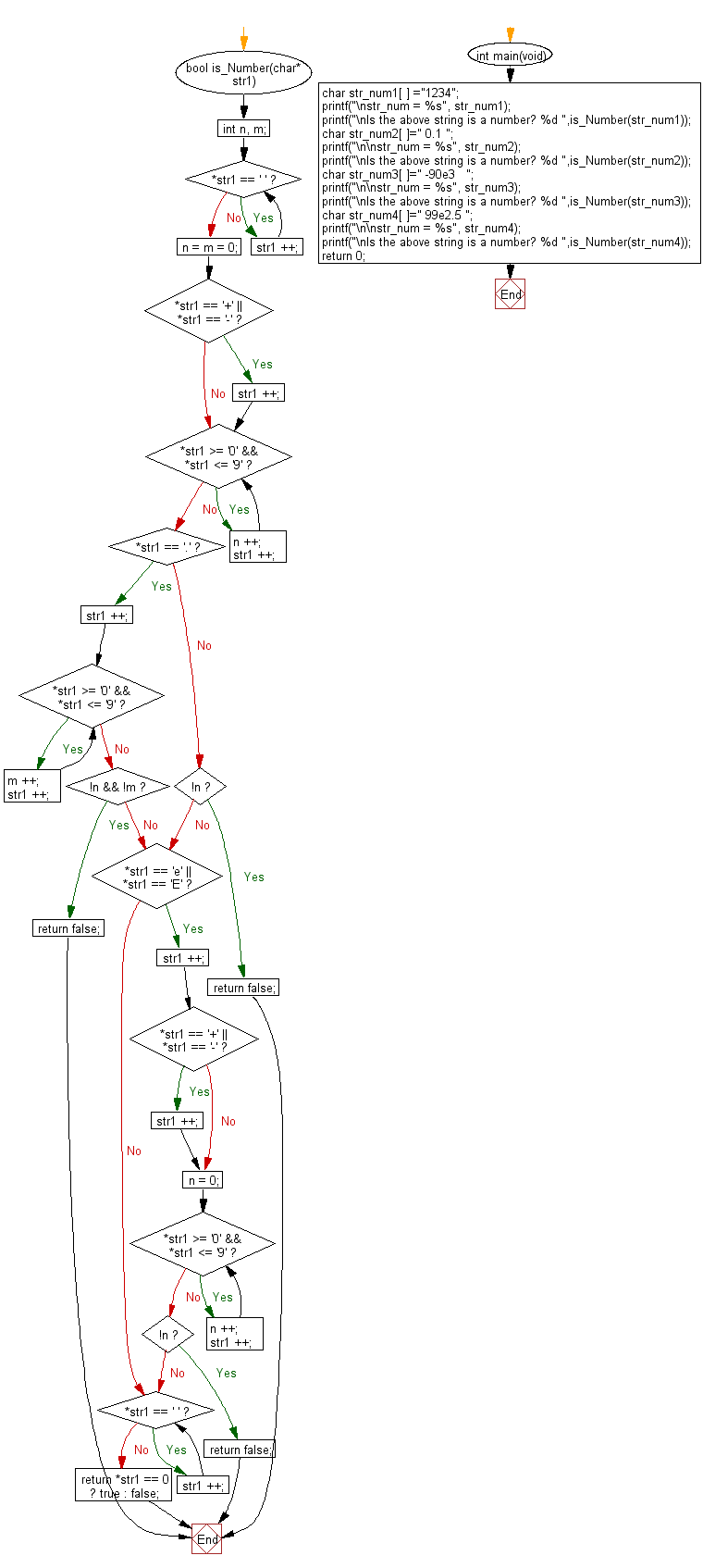
For more Practice: Solve these Related Problems:
- Write a C program to validate if a string represents a valid decimal number using state machine logic.
- Write a C program to check decimal validity by manually parsing the string character by character.
- Write a C program to determine if a string is a number, handling optional spaces, signs, and decimal points.
- Write a C program to validate a numeric string and flag errors if multiple decimal points or invalid characters are found.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to get the kth permutation sequence from two given integers n and k.
Next: Write a C program to get the fraction part from two given integers representing the numerator and denominator in string format.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.