C Exercises: Count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n
11. Count Digit 1 Occurrences Variants
Write a C program to count the total number of digits 1 appearing in all positive integers less than or equal to a given integer n.
For example:
Input n = 12,
Return 5, because digit 1 occurred 5 times in the following numbers: 1, 10, 11, 12.
Example:
Input:
n = 12
n = 30
Output:
Total number of digit 1 appearing in 12 (less than or equal) is 5.
Total number of digit 1 appearing in 30 (less than or equal) is 13.
Visual Presentation:
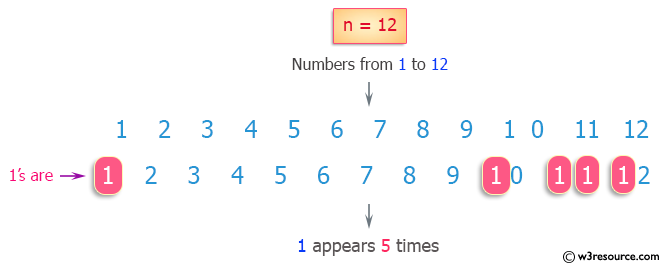
Sample Solution:
C Code:
#include <stdio.h>
// Function to count the total number of occurrences of digit 1 in numbers up to the given limit
static int count_DigitOne(int n) {
int m = 0, k = 0, x = 0, base = 1;
// Loop to iterate through each digit of the number
while (n > 0) {
k = n % 10; // Get the current digit
n = n / 10; // Update the number by removing the last digit
// Count occurrences of digit 1 based on the current digit
if (k > 1) {
x += (n + 1) * base; // Increment occurrences if the digit is greater than 1
} else if (k < 1) {
x += n * base; // Increment occurrences if the digit is less than 1
} else {
x += n * base + m + 1; // Increment occurrences if the digit is equal to 1
}
m += k * base; // Update the count of occurrences so far
base *= 10; // Update the base for the next digit
}
return x; // Return the total count of digit 1 occurrences
}
// Main function to test the count_DigitOne function with different values
int main(void) {
int n = 12;
printf("\nTotal number of digit 1 appearing in %d (less than or equal) is %d.", n, count_DigitOne(n));
n = 30;
printf("\nTotal number of digit 1 appearing in %d (less than or equal) is %d.", n, count_DigitOne(n));
return 0;
}
Sample Output:
Total number of digit 1 appearing in 12 (less than or equal) is 5. Total number of digit 1 appearing in 30 (less than or equal) is 13.
Flowchart:
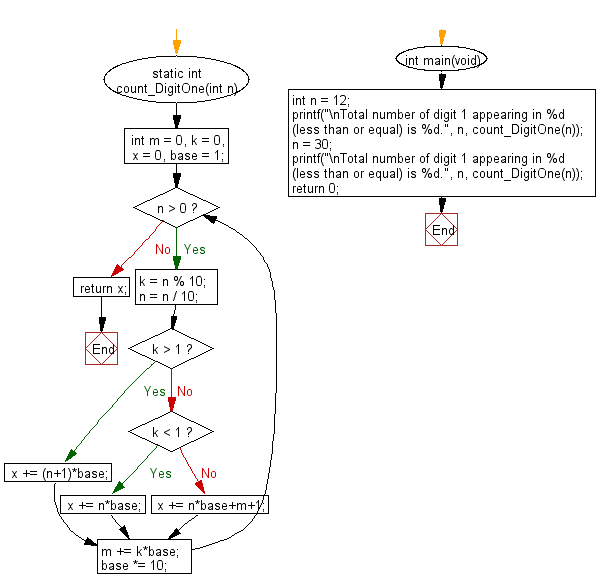
For more Practice: Solve these Related Problems:
- Write a C program to count the occurrences of digit 1 in all numbers from 1 to n using mathematical analysis.
- Write a C program to calculate the frequency of digit 1 without iterating through every number.
- Write a C program to count digit 1 occurrences recursively and compare with iterative results.
- Write a C program to generate a histogram of digit frequencies for numbers 1 to n and highlight digit 1.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to find the number of trailing zeroes in a given factorial.
Next: Write a C programming to add repeatedly all digits of a given non-negative number until the result has only one digit.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.