C Exercises: Add repeatedly all digits of a given non-negative number until the result has only one digit
C Programming Mathematics: Exercise-12 with Solution
Write a C program to add repeatedly all digits of a given non-negative number until the result has only one digit.
Example:
Input: 48
Output: 2
Explanation: The formula is like: 4 + 8 = 12, 1 + 2 = 3.
Visual Presentation:
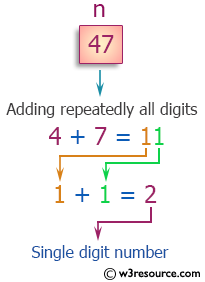
Sample Solution:
C Code:
#include <stdio.h>
// Function to calculate the digital root of a number (single-digit sum)
static int addDigits(int num) {
// Formula to calculate the digital root of a number using a mathematical property
// The digital root of a positive integer num is equivalent to num - (num - 1) / 9 * 9
return num - (num - 1) / 9 * 9;
}
// Main function to test the addDigits function with different numbers
int main(void) {
int n = 12;
printf("\nInitial number is %d, Single digit number is %d.", n, addDigits(n));
n = 47;
printf("\n\nInitial number is %d, Single digit number is %d.", n, addDigits(n));
return 0;
}
Sample Output:
Initial number is 12, Single digit number is 3. Initial number is 47, Single digit number is 2.
Flowchart:
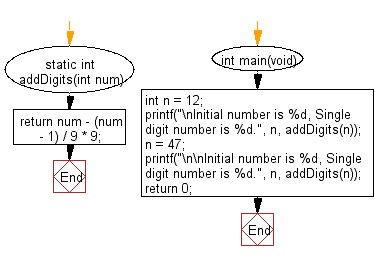
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C program to count the total number of digit 1 appearing in all positive integers less than or equal to a given integer n.
Next: Write a C programming to check if a given integer is a power of three.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/math/c-math-exercise-12.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics