C Exercises: Check if a given integer is a power of three
13. Power of Three Check Variants
Write a C program to check if a given integer is a power of three.
Example:
Input: 9
Output: true
Input: 81
Output: true
Input: 45
Output: false
Visual Presentation:
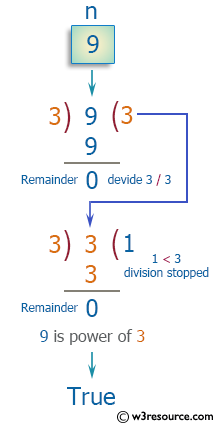
Sample Solution:
C Code:
#include <stdio.h>
#include <stdbool.h>
// Function to check if a number is a power of three
static bool is_PowerOf_Three(int n) {
#if 0 // Alternative approach using recursive function
if (n == 1) return true; // Base case: If n is 1, it is a power of three
if (n == 0 || n % 3) return false; // If n is not divisible by 3 or is 0, it is not a power of three
return is_PowerOf_Three(n / 3); // Recursively check if n divided by 3 is a power of three
#else // Optimized approach using mathematical property
return (n > 0 && (1162261467 % n) == 0); // Return true if n is greater than 0 and 1162261467 is divisible by n
#endif
}
// Main function to test the is_PowerOf_Three function with different numbers
int main(void) {
int n = 9;
printf("\nIf %d is a power of three? %d", n, is_PowerOf_Three(n));
n = 81;
printf("\n\nIf %d is a power of three? %d", n, is_PowerOf_Three(n));
n = 45;
printf("\n\nIf %d is a power of three? %d", n, is_PowerOf_Three(n));
return 0;
}
Sample Output:
If 9 is power of three? 1 If 81 is power of three? 1 If 45 is power of three? 0
Flowchart:
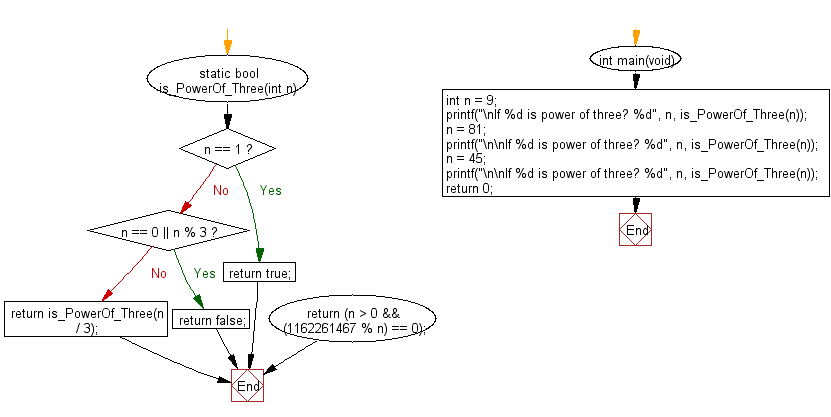
For more Practice: Solve these Related Problems:
- Write a C program to determine if an integer is a power of three using logarithmic functions.
- Write a C program to check if a number is a power of three by iterative division until reaching one.
- Write a C program to verify the power-of-three property without loops by using recursion.
- Write a C program to test the power of three condition while handling negative and zero inputs appropriately.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to add repeatedly all digits of a given non-negative number until the result has only one digit.
Next: Write a C programming to calculate the number of 1's in their binary representation and return them as an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.