C Exercises: Find the total number of full staircase rows that can be formed from given number of dices
18. Full Staircase Rows Variants
Write a C program to find the total number of full staircase rows that can be formed from a given number of dice.
staircase rows format :
Example 1:
n = 5
The dices can form the following rows:
As the 3rd row is incomplete the program will return 2 (full staircase rows).
Example 2:
n = 8
The dices can form the following rows:
As the 4th row is incomplete the program will return 3 (full staircase rows).
Sample Solution:
C Code:
#include <stdio.h>
// Function to check the total number of full staircase rows based on a given number of blocks 'n'
int check_stair_case(int n) {
if (n < 0) return -1; // Return -1 if the input 'n' is negative
if (n == 0) return 0; // Return 0 if the input 'n' is zero
int rows = 0; // Variable to keep track of the number of rows
long sum = 0; // Variable to store the sum of blocks in each row
// Loop to calculate the total number of full staircase rows based on 'n'
while (sum <= n) {
sum += rows + 1; // Increment the sum by the number of blocks in the next row
rows++; // Increment the row count
}
return rows - 1; // Return the total number of full staircase rows
}
// Main function to test the check_stair_case function with different values of 'n'
int main(void) {
int n = 5;
printf("Input number %d ", n);
printf("\nTotal number of full staircase rows are %d ", check_stair_case(n));
n = 8;
printf("\nInput number %d ", n);
printf("\nTotal number of full staircase rows are %d ", check_stair_case(n));
return 0;
}
Sample Output:
Input number 5 Total number of full staircase rows are 2 Input number 8 Total number of full staircase rows are 3
Flowchart:
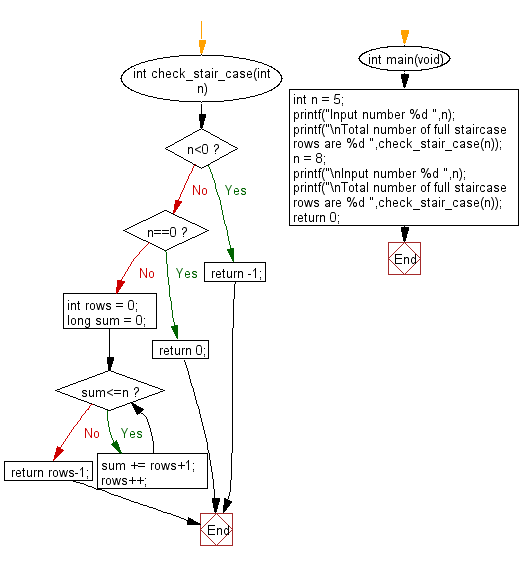
For more Practice: Solve these Related Problems:
- Write a C program to calculate the maximum number of full staircase rows that can be formed from a given number of dice using arithmetic series.
- Write a C program to simulate staircase row formation and count complete rows without overshooting.
- Write a C program to determine full staircase rows by subtracting successive row counts until insufficient dice remain.
- Write a C program to output each completed row and the remaining dice count after each row formation.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to find the nth digit of number 1 to n.
Next: Write a C program to find the square root of a number using Babylonian method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.