C Exercises: Find the nth digit of number 1 to n
17. Nth Digit in Sequence Variants
Write a C program to find the nth digit of the number 1 to n?
Infinite integer sequence: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12 .. where n is a positive integer.
Example:
Input:
7
Output:
7
Input:
12
Output:
1
The 12th digit of the sequence 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, ... is 1, which is part of the number 11.
Visual Presentation:
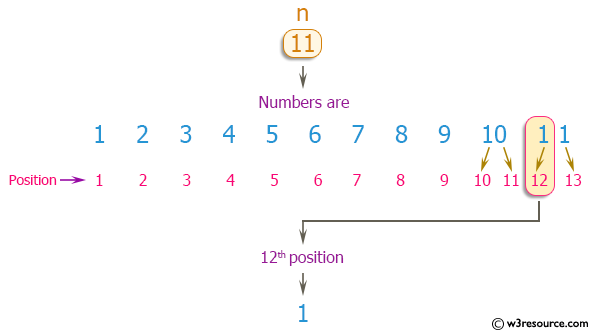
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the Nth digit in a sequence
int find_Nth_Digit(int n) {
unsigned int i, j, k;
i = j = 1;
// Loop to find the appropriate position of the digit in the sequence
while (n > 9 * i * j) {
n -= 9 * i * j; // Decrement n based on the sequence length
j *= 10; // Increase j to move to the next digit length
i++; // Increment i to keep track of digit length
}
k = j + (n - 1) / i; // Calculate the position of the digit in the sequence
// Loop to extract the desired digit
for (j = (n - 1) % i; j < i - 1; j++) {
k = k / 10; // Move to the specific digit position
}
return k % 10; // Return the Nth digit
}
// Main function to test the find_Nth_Digit function
int main(void) {
int n = 7;
printf("\n%d digit of the sequence is %d", n, find_Nth_Digit(n));
n = 12;
printf("\n%d digit of the sequence is %d", n, find_Nth_Digit(n));
return 0;
}
Sample Output:
7 digit of the sequence is 7 12 digit of the sequence is 1
Flowchart:
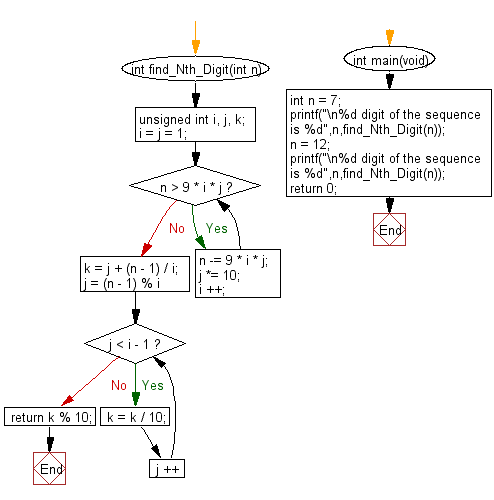
For more Practice: Solve these Related Problems:
- Write a C program to find the nth digit in the infinite sequence formed by concatenating all positive integers.
- Write a C program to calculate the nth digit by simulating the concatenation process without storing the full sequence.
- Write a C program to determine the nth digit using mathematical formulas to jump over digit groups.
- Write a C program to compute the nth digit and optimize for very large n using logarithmic estimations.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to print numbers from 1 to an given integer(N) in lexicographic order.
Next: Write a C programming to find the total number of full staircase rows that can be formed from given number of dices.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.