C Exercises: Print numbers from 1 to an given integer(N) in lexicographic order
16. Lexicographic Order Numbers Variants
Lexicographical order:
From Wikipedia,
In mathematics, the lexicographic or lexicographical order (also known as lexical order, dictionary order, alphabetical order or lexicographic(al) product) is a generalization of the way words are alphabetically ordered based on the alphabetical order of their component letters. This generalization consists primarily in defining a total order on the sequences (often called strings in computer science) of elements of a finite totally ordered set, often called an alphabet.
Write a C program to print numbers from 1 to an integer(N) in lexicographic order.
Example:
Input: 10
Output:
Print numbers from 1 to 10 in lexicographic order-
1 10 2 3 4 5 6 7 8 9
Input: 25
Output:
Print numbers from 1 to 25 in lexicographic order-
1 10 11 12 13 14 15 16 17 18 19 2 20 21 22 23 24 25 3 4 5 6 7 8 9
Visual Presentation:
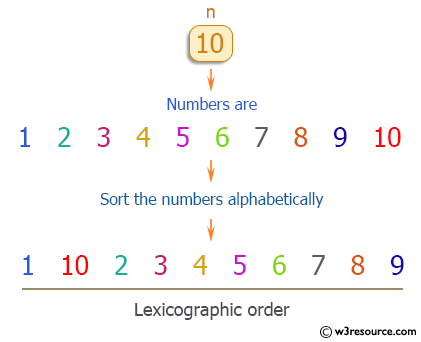
Sample Solution:
C Code:
#include <stdio.h> // Include standard input-output library
#include <math.h> // Include math library
#include <stdlib.h> // Include standard library
// Function to print numbers from 1 to n in lexicographic order
void print_lexicographic(int n)
{
int m, j, i = 1; // Declare variables m, j, and i with initialization
printf("\n\nPrint numbers from 1 to %d in lexicographic order-\n",n); // Print message indicating the start of lexicographic printing
while(i<= 9){ // While loop to iterate over digits 1 to 9
j = 1; // Initialize j to 1
while( j <= n){ // Nested while loop to iterate over numbers less than or equal to n
m = 0; // Initialize m to 0
while(m < j) { // Nested while loop to iterate over digits 0 to j-1
if((m + j * i)<= n){ // Check if the number formed is less than or equal to n
printf("%d ", m + j * i); // Print the number
}
m=m+1; // Increment m
}
j= j*10; // Update j to the next power of 10
}
i=i+1; // Increment i
}
}
int main(void)
{
print_lexicographic(10); // Call function to print lexicographic numbers from 1 to 10
print_lexicographic(25); // Call function to print lexicographic numbers from 1 to 25
print_lexicographic(40); // Call function to print lexicographic numbers from 1 to 40
print_lexicographic(100); // Call function to print lexicographic numbers from 1 to 100
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Print numbers from 1 to 10 in lexicographic order- 1 10 2 3 4 5 6 7 8 9 Print numbers from 1 to 25 in lexicographic order- 1 10 11 12 13 14 15 16 17 18 19 2 20 21 22 23 24 25 3 4 5 6 7 8 9 Print numbers from 1 to 40 in lexicographic order- 1 10 11 12 13 14 15 16 17 18 19 2 20 21 22 23 24 25 26 27 28 29 3 30 31 32 33 34 35 36 37 38 39 4 40 5 6 7 8 9 Print numbers from 1 to 100 in lexicographic order- 1 10 11 12 13 14 15 16 17 18 19 100 2 20 21 22 23 24 25 26 27 28 29 3 30 31 32 33 34 35 36 37 38 39 4 40 41 42 43 44 45 46 47 48 49 5 50 51 52 53 54 55 56 57 58 59 6 60 61 62 63 64 65 66 67 68 69 7 70 71 72 73 74 75 76 77 78 79 8 80 81 82 83 84 85 86 87 88 89 9 90 91 92 93 94 95 96 97 98 99
Flowchart:
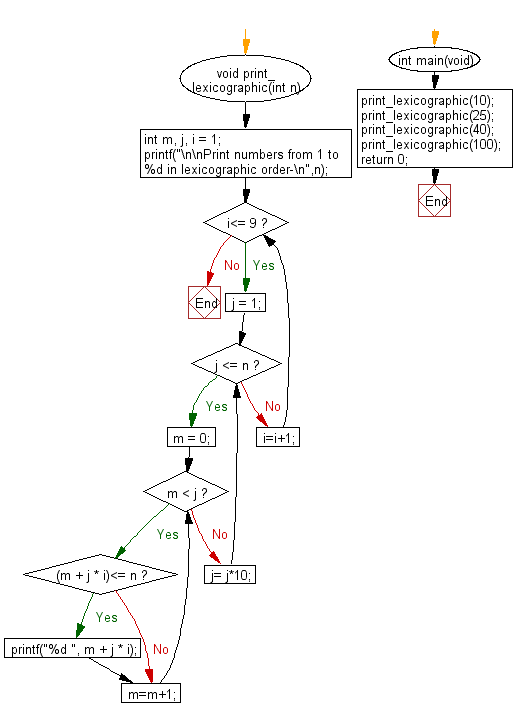
For more Practice: Solve these Related Problems:
- Write a C program to print numbers from 1 to N in lexicographical order using recursion.
- Write a C program to generate lexicographic order of numbers by simulating a depth-first search on number strings.
- Write a C program to sort the numbers from 1 to N lexicographically without converting them to strings.
- Write a C program to display lexicographic order of numbers and compare with numerical order.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to get the maximum product from a given integer after breaking the integer into the sum of at least two positive integers.
Next: Write a C programming to find the nth digit of number 1 to n?.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.