C Exercises: Get the maximum product from a given integer after breaking the integer into the sum of at least two positive integers
15. Maximum Product After Integer Break Variants
Write a C program to get the maximum product of a given integer after breaking the integer into the sum of at least two positive integers.
Example:
Input: 12
Output: 81
Explanation: 12 = 3 + 3 + 3 + 3, 3 x 3 × 3 × 3 = 81.
Input: 7
Output: 12
Explanation: 7 = 3 + 2 + 2, 3 x 2 x 2 = 12.
Visual Presentation:
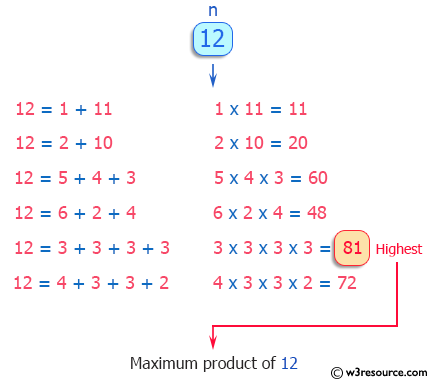
Sample Solution:
C Code:
#include <stdio.h> // Including standard input-output library
#include <math.h> // Including math library for pow function
#include <stdlib.h> // Including standard library for exit function
// Function to calculate the maximum product after breaking the integer
int integer_Break(int n) {
if (n == 2) { // If n is equal to 2
return 1; // Return 1 as maximum product
} else if (n == 3) { // If n is equal to 3
return 2; // Return 2 as maximum product
} else if (n % 3 == 0) { // If n is divisible by 3
return (int) pow(3, n / 3); // Calculate maximum product using pow function
} else if (n % 3 == 1) { // If n leaves remainder 1 when divided by 3
return 2 * 2 * (int) pow(3, (n - 4) / 3); // Calculate maximum product
} else { // If n leaves remainder 2 when divided by 3
return 2 * (int) pow(3, n / 3); // Calculate maximum product
}
}
// Main function
int main(void)
{
int n = 12; // Declare and initialize integer variable n with value 12
printf("\nMaximum product of %d after breaking the integer %d ", n, integer_Break(n)); // Print the maximum product
n = 7; // Assign value 7 to n
printf("\nMaximum product of %d after breaking the integer %d ", n, integer_Break(n)); // Print the maximum product
return 0; // Return 0 to indicate successful termination
}
Sample Output:
Maximum product of 12 after breaking the integer 81 Maximum product of 7 after breaking the integer 12
Flowchart:
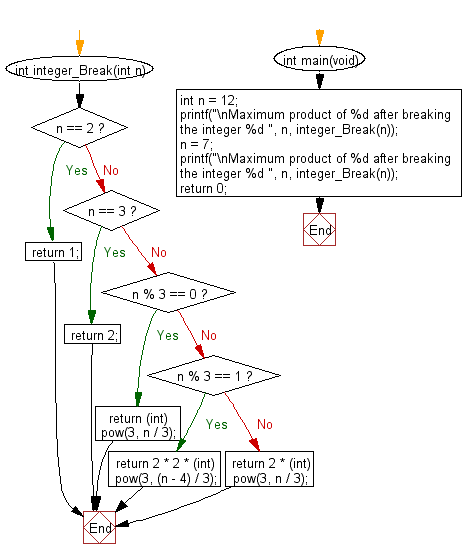
For more Practice: Solve these Related Problems:
- Write a C program to break an integer into at least two parts such that the product of the parts is maximized, using recursion.
- Write a C program to compute the maximum product after integer break by applying dynamic programming.
- Write a C program to compare the product obtained by greedy splitting versus optimal splitting for an integer.
- Write a C program to calculate the maximum product with a memoized solution and display intermediate splits.
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: Write a C programming to calculate the number of 1's in their binary representation and return them as an array.
Next: Write a C programming to print numbers from 1 to an given integer(N) in lexicographic order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.