C Exercises: Index of first presence of a string in other
14. Find First Occurrence of Substring Variants
Write a C programming to find the index of the first occurrence of a given string within another given string. If not found return -1.
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int sub_str_func(char *main_str, char *sub_str)
{
if (main_str == NULL || sub_str == NULL) {
return -1;
}
int main_len = strlen(main_str);
int sub_len = strlen(sub_str);
if (main_len < sub_len) {
return -1;
}
if (sub_len == 0) {
return 0;
}
int i, j;
int bad_steps[128];
for (i = 0; i < 128; i++) {
bad_steps[i] = sub_len;
}
for (i = 0; i < sub_len; i++) {
bad_steps[sub_str[i]] = sub_len - 1 - i;
}
int *good_steps = malloc(sub_len * sizeof(int));
for (i = 0; i < sub_len; i++) {
good_steps[i] = sub_len;
for (j = i - 1; j >= 0; j--) {
if (!memcmp(sub_str + i, sub_str + j, sub_len - i)) {
good_steps[i] = i - j;
break;
}
}
}
char *p = main_str + sub_len - 1;
char *q = sub_str + sub_len - 1;
char *r = p;
while (p - main_str < main_len) {
int step = 0;
for (i = 1; i <= sub_len && *p == *q; i++) {
if (q == sub_str) {
return p - main_str;
}
if (good_steps[sub_len - i] > step) {
step = good_steps[sub_len - i];
}
p--;
q--;
}
if (i == 1 && bad_steps[*p] > step) {
step = bad_steps[*p];
}
r += step;
p = r;
q = sub_str + sub_len - 1;
}
return -1;
}
static int strStr(char *main_str, char *sub_str)
{
unsigned int main_len = strlen(main_str);
unsigned int sub_len = strlen(sub_str);
if (sub_len == 0) {
return 0;
}
int i, j;
for (i = 0; i < main_len; i++) {
int found = 1;
if (main_str[i] == sub_str[0]) {
for (j = 1; j < sub_len; j++) {
if (i + j < main_len) {
if (main_str[i + j] != sub_str[j]) {
found = 0;
break;
}
} else {
return -1;
}
}
if (found) {
return i;
}
}
}
return -1;
}
int main(void)
{
char main_str[] = "w3resource.com";
char sub_str[] = "source";
printf("\nMain string: %s",main_str);
printf("\nSubstring searched in main string: %s",sub_str);
printf("\nStarting position of the substring in the main string: %d", sub_str_func(main_str, sub_str));
return 0;
}
Sample Output:
Main string: w3resource.com Substring searched in main string: source Starting position of the substring in the main string: 4
Pictorial Presentation:
Flowchart: 1
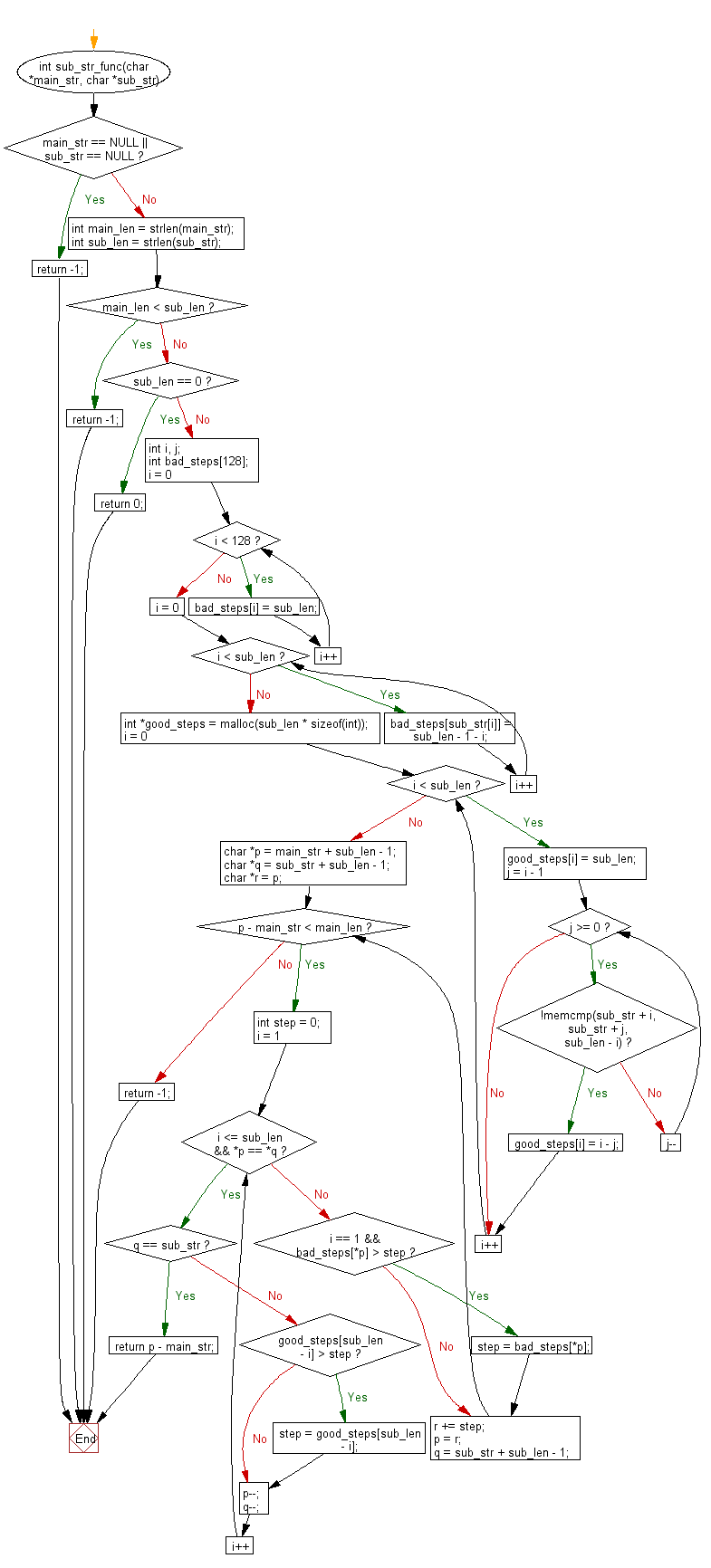
Flowchart: 2
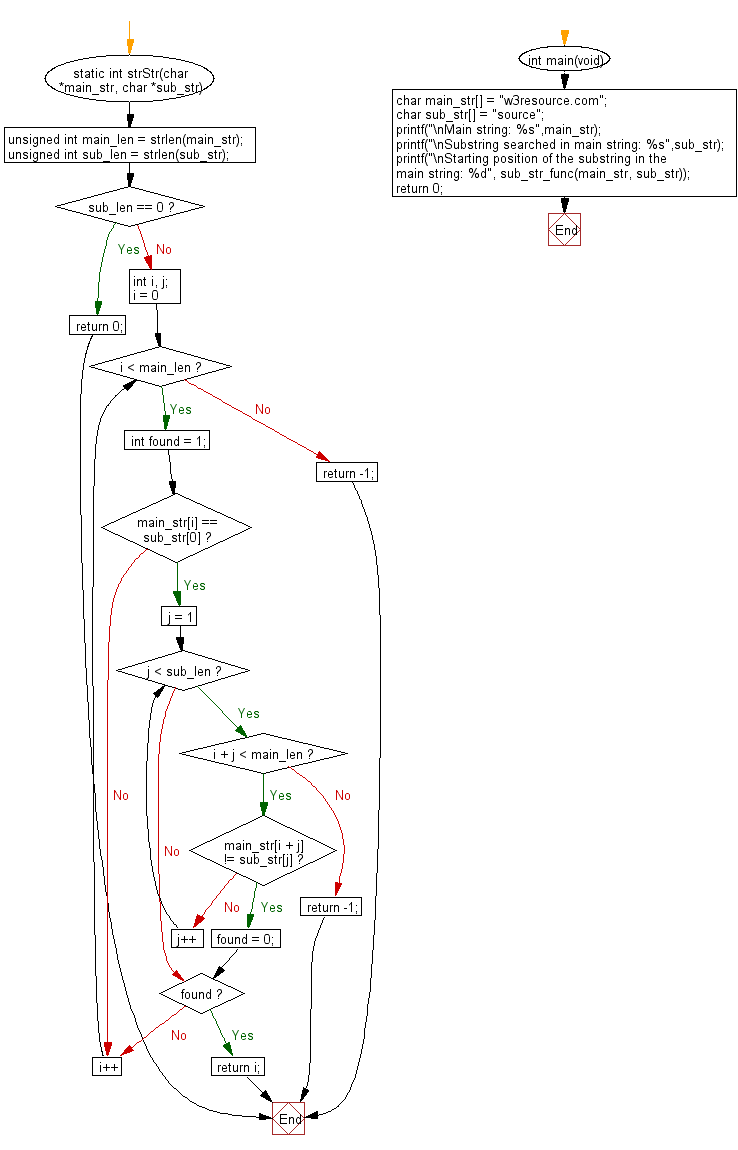
For more Practice: Solve these Related Problems:
- Write a C program to find the index of the first occurrence of a substring using the Knuth-Morris-Pratt algorithm.
- Write a C program to search for a substring using a naive approach and return all starting indices.
- Write a C program to perform a case-insensitive substring search and return the starting index.
- Write a C program to find the first occurrence of a substring without using library functions like strstr.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Array length, remove instances of a valueArray length, remove instances of a value.
Next C Programming Exercise: Divide two integers without multiply, divide, modulating.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.