C Exercises: Divide two integers without multiply, divide, modulating
15. Divide Integers Without Multiplication/Division/Mod Variants
Write a C program to divide two given integers without using the multiplication, division and mod operator. Return the quotient after dividing.
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
int divide_two(int dividend_num, int divisor_num)
{
int sign = (float) dividend_num / divisor_num > 0 ? 1 : -1;
unsigned int dvd = dividend_num > 0 ? dividend_num : -dividend_num;
unsigned int dvs = divisor_num > 0 ? divisor_num : -divisor_num;
unsigned int bit_num[33];
unsigned int i = 0;
long long d = dvs;
bit_num[i] = d;
while (d <= dvd) {
bit_num[++i] = d = d << 1;
}
i--;
unsigned int result = 0;
while (dvd >= dvs) {
if (dvd >= bit_num[i]) {
dvd -= bit_num[i];
result += (1<<i);
} else {
i--;
}
}
if (result > INT_MAX && sign > 0) {
return INT_MAX;
}
return (int) result * sign;
}
int main(void)
{
int dividend_num = 15;
int divisor_num = 3;
printf("Quotient after dividing %d and %d : %d", dividend_num, divisor_num, divide_two(dividend_num, divisor_num));
return 0;
}
Sample Output:
Quotient after dividing 15 and 3 : 5
Pictorial Presentation:
Flowchart:
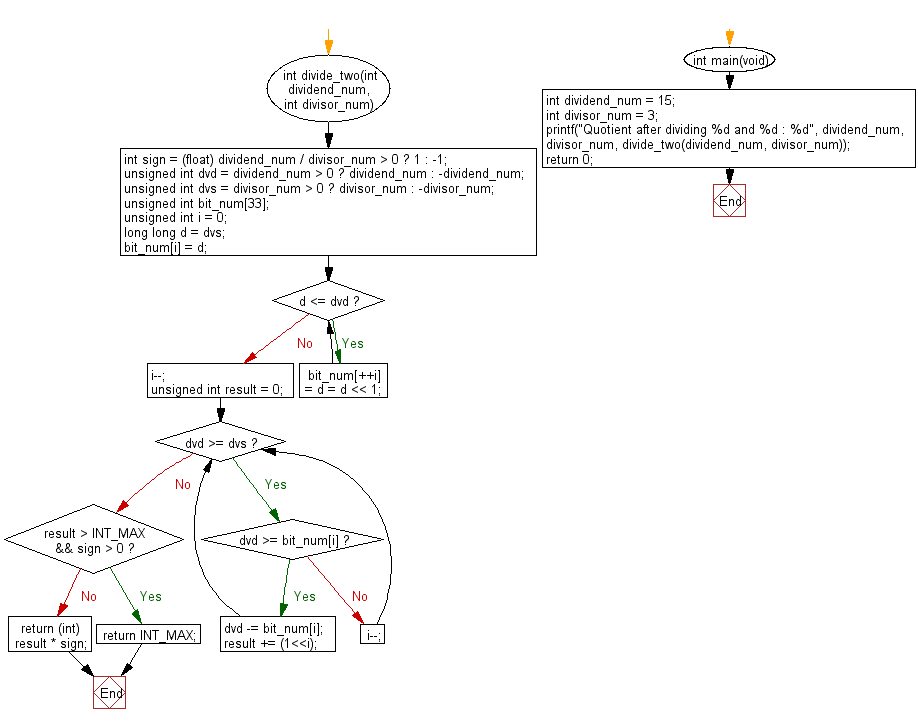
For more Practice: Solve these Related Problems:
- Write a C program to perform integer division using repeated subtraction and recursion.
- Write a C program to simulate division by using bit shifting and subtraction only.
- Write a C program to compute the quotient and remainder of two integers via iterative subtraction.
- Write a C program to implement division using a recursive approach without multiplication, division, or modulus operators.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Index of first presence of a string in other.
Next C Programming Exercise: Length, longest valid parentheses substring.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.