C++ String Exercises: Reverse the words in a string that have odd lengths
35. Reverse Words with Odd Lengths in a String
Write a C++ program to reverse all words that have odd lengths in a string.
Test Data:
("Exercises Practice Solution" ) -> "sesicrexE Practice Solution"
("The quick brown fox jumps over the lazy dog") -> "ehT kciuq nworb xof spmuj over eht lazy dog."
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
// Function to reverse words with odd lengths in the input string 's'
string test(string s) {
stringstream str(s); // Create a stringstream object 'str' with string 's'
string temp, result(""); // Declare strings to store temporary values and the final result
// Loop through the words separated by spaces in the stringstream
while (getline(str, temp, ' ')) {
// Check if the length of the word is odd (using bitwise AND operation)
if ((temp.length() & 1) == 1)
reverse(temp.begin(), temp.end()); // Reverse the word if its length is odd
result += temp + " "; // Append the word to the result string with a space
}
result.pop_back(); // Remove the extra space at the end
return result; // Return the modified string
}
int main() {
// Test cases
string text = "Exercises Practice Solution";
cout << "String: " << text;
cout << "\nReverse the words in the said string that have odd lengths:\n";
cout << test(text) << endl; // Display the modified string
text = "The quick brown fox jumps over the lazy dog.";
cout << "\nString: " << text;
cout << "\nReverse the words in the said string that have odd lengths:\n";
cout << test(text) << endl; // Display the modified string
}
Sample Output:
String: Exercises Practice Solution Reverse the words in the said string that have odd lengths: sesicrexE Practice Solution String: The quick brown fox jumps over the lazy dog. Reverse the words in the said string that have odd lengths: ehT kciuq nworb xof spmuj over eht lazy dog.
Flowchart:
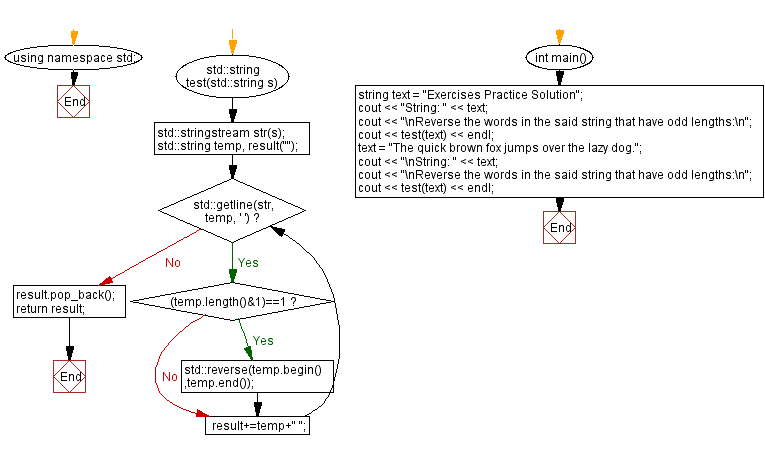
For more Practice: Solve these Related Problems:
- Write a C++ program to reverse only the words in a sentence that have an odd number of characters.
- Write a C++ program that processes a string and outputs a modified string where every word with an odd length is reversed.
- Write a C++ program to scan a sentence and reverse words of odd length using a loop and string reversal function.
- Write a C++ program that reads a sentence, reverses only odd-length words, and prints the updated sentence while preserving spaces.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Remove a word from a given string.
Next C++ Exercise: Check two consecutive, identical letters in a word.What is the difficulty level of this exercise?