C++ String Exercises: Check two consecutive, identical letters in a word
36. Check for Two Consecutive Identical Letters
Write a C++ program to check whether there are two consecutive (following each other continuously), identical letters in a given string.
Test Data:
("Exercises") -> 0
("Yellow") -> 1
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Include all standard libraries
using namespace std; // Using the standard namespace
// Function to check if there are two consecutive identical letters in the given word
bool test(string word) {
for (int i = 0; i < word.size() - 1; i++) { // Loop through the word's characters
if (word[i] == word[i + 1]) { // Check if current character is equal to the next character
return true; // Return true if two consecutive identical letters are found
}
}
return false; // Return false if no consecutive identical letters are found
}
int main() {
// Test cases
string text = "Exercises";
cout << "Word: " << text;
cout << "\nIf there are two consecutive identical letters in the said string?\n";
cout << test(text) << endl; // Display the result of the test for the word "Exercises"
text = "Yellow";
cout << "\nWord: " << text;
cout << "\nIf there are two consecutive identical letters in the said string?\n";
cout << test(text) << endl; // Display the result of the test for the word "Yellow"
}
Sample Output:
Word: Exercises If there are two consecutive identical letters in the said string? 0 Word: Yellow If there are two consecutive identical letters in the said string? 1
Flowchart:
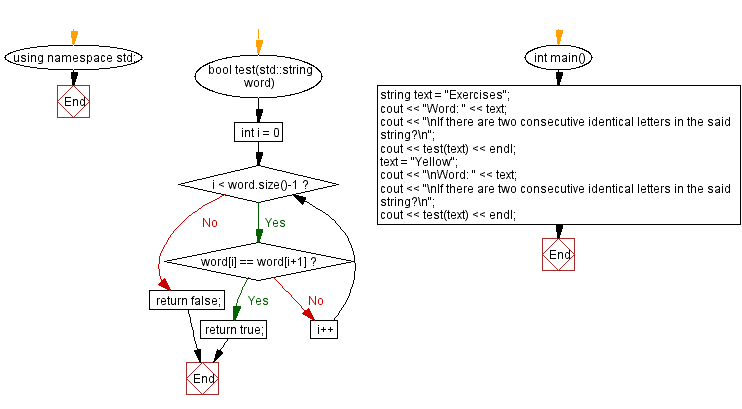
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a string contains any two adjacent identical letters and return a boolean.
- Write a C++ program that reads a string and checks for any occurrence of repeated consecutive characters.
- Write a C++ program to scan a string for double letters and output 1 if found, otherwise 0.
- Write a C++ program that iterates over a string and prints true if any two letters in succession are the same.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reverse the words in a string that have odd lengths.
Next C++ Exercise: Count occurrences of a certain character in a string.What is the difficulty level of this exercise?