C++ String Exercises: Remove a word from a given string
34. Remove a Specific Word from a String
Write a C++ program that removes a specific word from a given string. Return the updated string.
Test Data:
("Exercises Practice Solution", "Solution") -> "Exercises Practice"
("Exercises Practice Solution", "Practice ") -> "Exercises Solution"
("Exercises Practice Solution", " Solution") -> " Practice Solution"
Sample Solution:
C++ Code:
#include <bits/stdc++.h> // Includes all standard libraries
using namespace std; // Using the standard namespace
// Function to remove occurrences of 'w' from 's'
string test(string s, string w) {
// While 'w' is found in 's', replace it with an empty string
while (s.find(w) != string::npos)
s.replace(s.find(w), w.length(), "");
return s; // Return the modified string 's'
}
int main() {
string text = "Exercises Practice Solution"; // Original string
string word = "Solution"; // Word to be removed from 'text'
cout << "String: " << text; // Display original string
cout << "\nWord to remove: " << word; // Display word to be removed
cout << "\nNew string, after removing the said word:\n";
cout << test(text, word) << endl; // Call 'test' function and display modified string
// Repeat the same process for different words to be removed
word = "Practice";
cout << "\nString: " << text;
cout << "\nWord to remove: " << word;
cout << "\nNew string, after removing the said word:\n";
cout << test(text, word) << endl;
word = "Exercises";
cout << "\nString: " << text;
cout << "\nWord to remove: " << word;
cout << "\nNew string, after removing the said word:\n";
cout << test(text, word) << endl;
// The main function displays original strings, words to be removed,
// and the resulting strings after removing those words.
}
Sample Output:
String: Exercises Practice Solution Word to remove: Solution New string, after removing the said word: Exercises Practice String: Exercises Practice Solution Word to remove: Practice New string, after removing the said word: Exercises Solution String: Exercises Practice Solution Word to remove: Exercises New string, after removing the said word: Practice Solution
Flowchart:
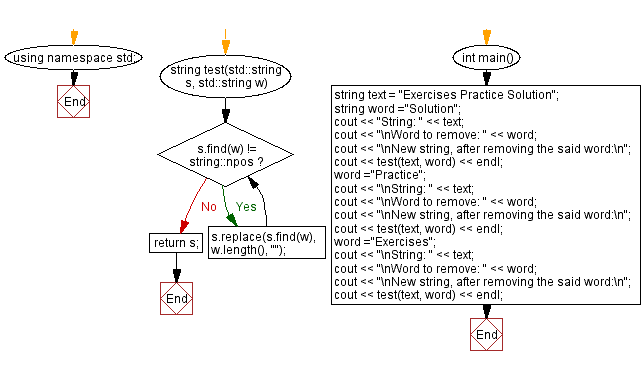
For more Practice: Solve these Related Problems:
- Write a C++ program to remove all instances of a given word from a sentence and output the modified string.
- Write a C++ program that reads a sentence and a target word, then deletes the target word wherever it appears.
- Write a C++ program to search for a specific word in a string and remove it, adjusting spaces appropriately.
- Write a C++ program to process a string and replace a designated word with an empty string, then print the result.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Check first string contains letters from the second.
Next C++ Exercise: Reverse the words in a string that have odd lengths.What is the difficulty level of this exercise?