C#: Check the numbers of a given array are consecutive or not
Write a C# Sharp program to re-arrange the elements in a given array of numbers and check whether the numbers are consecutive or not.
From Wikipedia,
Consecutive numbers are numbers that follow each other in order. They have a difference of 1 between every two numbers. In a set of consecutive numbers, the mean and the median are equal.
If n is a number, then the next numbers will be n+1 and n+2.
Examples:
Consecutive numbers that follow each other in order:
- 1, 2, 3, 4, 5
- -3, −2, −1, 0, 1, 2, 3, 4
- 6, 7, 8, 9, 10, 11, 12, 13
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers with both positive and negative values
int[] nums = { -10, -11, -12, -13, -14, 15, 16, 17, 18, 19, 20 };
// Displaying original array elements
Console.WriteLine("Original array elements:");
for (int i = 0; i < nums.Length; i++)
{
Console.Write(nums[i] + " ");
}
// Checking if the numbers in the array are consecutive or not and displaying the result
Console.WriteLine("\nCheck if the numbers of the said array are consecutive or not! " + test(nums));
// Creating another array with both positive and negative integers
int[] nums1 = { -1, -2, -3, 0, 1, 4, 3, 2 };
Console.WriteLine("\nOriginal array elements:");
for (int i = 0; i < nums1.Length; i++)
{
Console.Write(nums1[i] + " ");
}
// Checking if the numbers in the second array are consecutive or not and displaying the result
Console.WriteLine("\nCheck if the numbers of the said array are consecutive or not! " + test(nums1));
}
// Method to check if the numbers in the array are consecutive or not
public static bool test(int[] nums)
{
// Sorting the array in ascending order
Array.Sort(nums);
// Looping through the sorted array to check if the numbers are consecutive
for (int i = 1; i < nums.Length; i++)
{
if (nums[i] - nums[i - 1] != 1)
{
// If the difference between adjacent numbers is not 1, return false
return false;
}
}
// If all numbers are consecutive, return true
return true;
}
}
}
Sample Output:
Original array elements: -10 -11 -12 -13 -14 15 16 17 18 19 20 Check the numbers of the said array are consecutive or not! False Original array elements: -1 -2 -3 0 1 4 3 2 Check the numbers of the said array are consecutive or not! True
Flowchart:
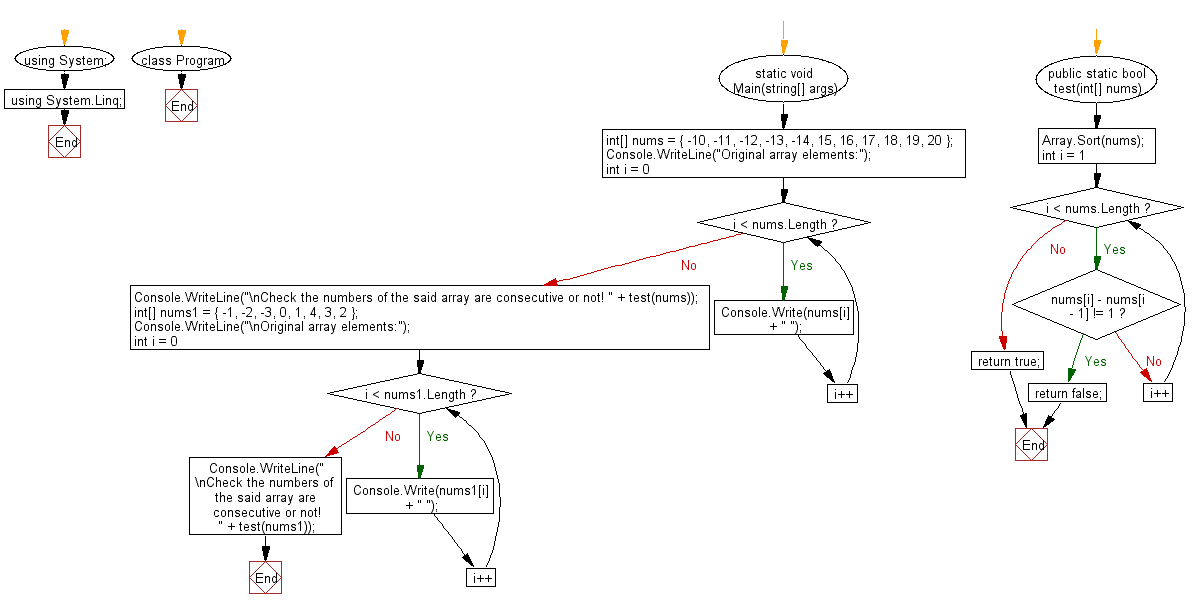
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous C# Sharp: Calculate the sum of two lowest negative numbers of a given array of integers.
Next C# Sharp: Smallest gap between the numbers in an array
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.