C#: Smallest gap between the numbers in an array
Write a C# Sharp program that calculates the smallest gap between numbers in an array of integers.
Sample Data:
({ 7, 5, 8, 9, 11, 23, 18 }) -> 1
({ 200, 300, 250, 151, 162 }) -> 11
Sample Solution-1:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums = { 7, 5, 8, 9, 11, 23, 18 };
// Displaying original array elements using string.Join method
Console.WriteLine("Original array elements:");
Console.WriteLine($"{string.Join(", ", nums)}");
// Finding and displaying the smallest gap between the numbers in the array
Console.WriteLine("Smallest gap between the numbers in the said array: " + test(nums));
// Creating another array of integers
int[] nums1 = { 200, 300, 250, 151, 162 };
Console.WriteLine("\nOriginal array elements:");
Console.WriteLine($"{string.Join(", ", nums1)}");
// Finding and displaying the smallest gap between the numbers in the second array
Console.WriteLine("Smallest gap between the numbers in the said array: " + test(nums1));
}
// Method to find the smallest gap between numbers in an array
public static int test(int[] nums)
{
// Sorting the array in ascending order
Array.Sort(nums);
// Using LINQ to calculate the differences between adjacent numbers and finding the minimum difference
return nums.Skip(1)
.Select((e, el) => e - nums[el])
.Min();
}
}
}
Sample Output:
Original array elements: 7, 5, 8, 9, 11, 23, 18 Smallest gap between the numbers in the said array: 1 Original array elements: 200, 300, 250, 151, 162 Smallest gap between the numbers in the said array: 11
Flowchart:
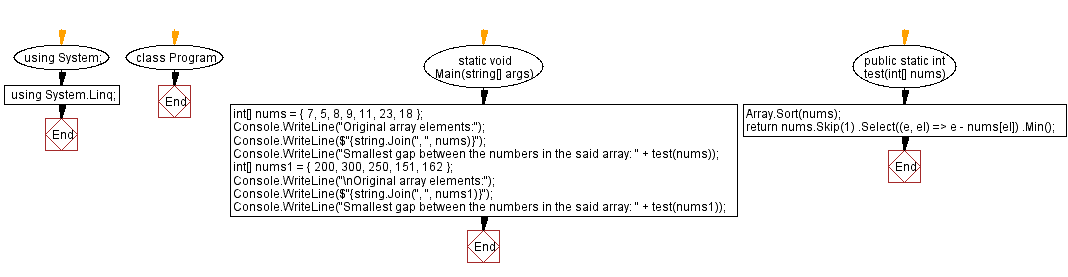
Sample Solution-2:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers
int[] nums = { 7, 5, 8, 9, 11, 23, 18 };
// Displaying original array elements using string.Join method
Console.WriteLine("Original array elements:");
Console.WriteLine($"{string.Join(", ", nums)}");
// Finding and displaying the smallest gap between the numbers in the array
Console.WriteLine("Smallest gap between the numbers in the said array: " + test(nums));
// Creating another array of integers
int[] nums1 = { 200, 300, 250, 151, 162 };
Console.WriteLine("\nOriginal array elements:");
Console.WriteLine($"{string.Join(", ", nums1)}");
// Finding and displaying the smallest gap between the numbers in the second array
Console.WriteLine("Smallest gap between the numbers in the said array: " + test(nums1));
}
// Method to find the smallest gap between numbers in an array
public static int test(int[] nums)
{
// Initializing a list to store differences between adjacent numbers
List<int> result = new List<int>();
// Sorting the array in ascending order
Array.Sort(nums);
// Iterating through the sorted array to calculate differences between adjacent numbers
for (int i = 0; i < nums.Length; i++)
{
// Checking if the current number is not the last element in the sorted array
if (nums[i] != nums.Last())
{
// Calculating and storing the difference between the current and next numbers
result.Add(nums[i + 1] - nums[i]);
}
}
// Returning the minimum difference calculated
return result.Min();
}
}
}
Sample Output:
Original array elements: 7, 5, 8, 9, 11, 23, 18 Smallest gap between the numbers in the said array: 1 Original array elements: 200, 300, 250, 151, 162 Smallest gap between the numbers in the said array: 11
Flowchart:
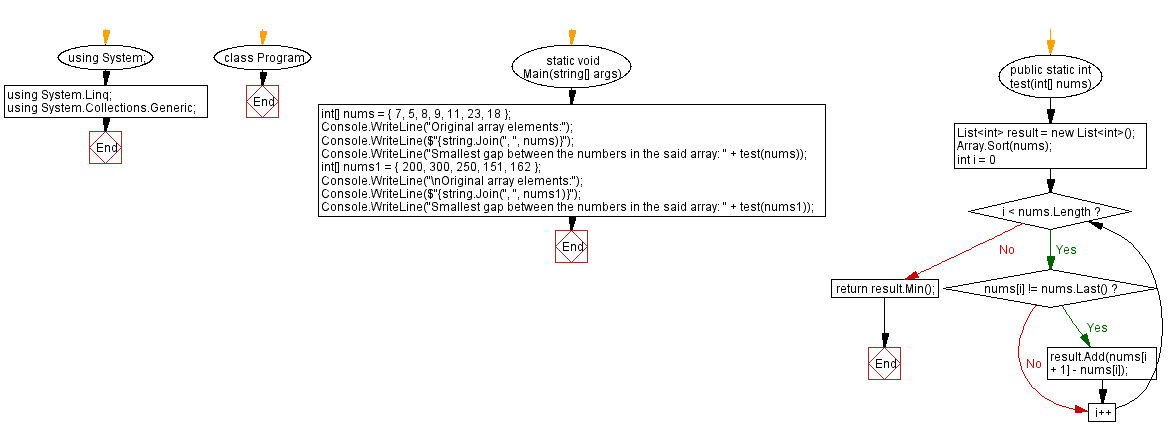
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous C# Sharp Exercise: Check the numbers of a array are consecutive or not.
Next C# Sharp Exercise: Check specific digit in an array of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.