C#: Sum of two lowest negative numbers of a given array of integers
Write a C# Sharp program to calculate the sum of the two lowest negative numbers in a given array of integers.
An integer (from the Latin integer meaning "whole") is colloquially defined as a number that can be written without a fractional component. For example, 21, 4, 0, and −2048 are integers,
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Initializing an array of integers with negative and positive values
int[] nums = { -10, -11, -12, -13, -14, 15, 16, 17, 18, 19, 20 };
// Displaying original array elements
Console.WriteLine("Original array elements:");
for (int i = 0; i < nums.Length; i++)
{
Console.Write(nums[i] + " ");
}
// Finding and displaying the sum of the two lowest negative numbers in the array
Console.WriteLine("\nSum of two lowest negative numbers of the said array of integers: " + test(nums));
// Creating another array with both negative and non-negative integers
int[] nums1 = { -1, -2, -4, 0, 3, 4 , 5, 9 };
Console.WriteLine("\nOriginal array elements:");
for (int i = 0; i < nums1.Length; i++)
{
Console.Write(nums1[i] + " ");
}
// Finding and displaying the sum of the two lowest negative numbers in the second array
Console.WriteLine("\nSum of two lowest negative numbers of the said array of integers: " + test(nums1));
}
// Method to find the sum of the two lowest negative numbers in an array
public static int test(int[] values)
{
// Using LINQ to filter negative numbers, sort them, take the two lowest values, and calculate their sum
return values.Where(x => x < 0).OrderBy(x => x).Take(2).Sum();
}
}
}
Sample Output:
Original array elements: -10 -11 -12 -13 -14 15 16 17 18 19 20 Sum of two lowest negative numbers of the said array of integers: -27 Original array elements: -1 -2 -4 0 3 4 5 9 Sum of two lowest negative numbers of the said array of integers: -6
Flowchart:
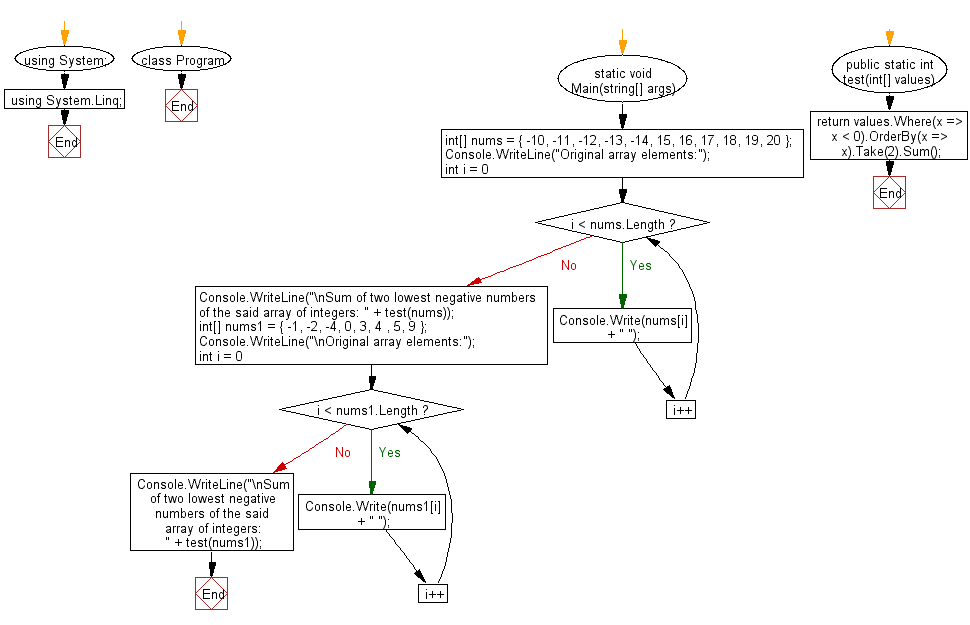
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous:Write a C# Sharp program to find the missing number in a given array of numbers between 10 and 20.
Next: Write a C# Sharp program to re-arrange the elements in a given array of numbers and check the numbers are consecutive or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.