Java: Abstract Vehicle Class with Car and Motorcycle Subclasses
Java Abstract Class: Exercise-7 with Solution
Write a Java program to create an abstract class Vehicle with abstract methods startEngine() and stopEngine(). Create subclasses Car and Motorcycle that extend the Vehicle class and implement the respective methods to start and stop the engines for each vehicle type.
Sample Solution:
Java Code:
// Vehicle.java
// Abstract class Vehicle
abstract class Vehicle {
// Abstract method to start the engine
public abstract void startEngine();
// Abstract method to stop the engine
public abstract void stopEngine();
}
// Car.java
// Class Car extending Vehicle
class Car extends Vehicle {
// Overriding the startEngine method
@Override
public void startEngine() {
// Printing message to indicate engine start
System.out.println("Car: Starting the engine...");
}
// Overriding the stopEngine method
@Override
public void stopEngine() {
// Printing message to indicate engine stop
System.out.println("Car: Stopping the engine...");
}
}
// Motorcycle.java
// Class Motorcycle extending Vehicle
class Motorcycle extends Vehicle {
// Overriding the startEngine method
@Override
public void startEngine() {
// Printing message to indicate engine start
System.out.println("Motorcycle: Starting the engine...");
}
// Overriding the stopEngine method
@Override
public void stopEngine() {
// Printing message to indicate engine stop
System.out.println("Motorcycle: Stopping the engine...");
}
}
//Main.java
public class Main {
// The main method, the entry point of the program
public static void main(String[] args) {
// Create an instance of Car as a Vehicle
Vehicle car = new Car();
// Create an instance of Motorcycle as a Vehicle
Vehicle motorcycle = new Motorcycle();
// Call the startEngine method on the car object
car.startEngine();
// Call the stopEngine method on the car object
car.stopEngine();
// Call the startEngine method on the motorcycle object
motorcycle.startEngine();
// Call the stopEngine method on the motorcycle object
motorcycle.stopEngine();
}
}
Output:
Car: Starting the engine... Car: Stopping the engine... Motorcycle: Starting the engine... Motorcycle: Stopping the engine...
Explanation:
In the above exercise -
- The abstract class "Vehicle" has two abstract methods: startEngine() and stopEngine(). The subclasses Car and Motorcycle extend the "Vehicle" class and provide their own implementations for these abstract methods.
- The "Car" class starts and stops the car's engine, while the Motorcycle class starts and stops the motorcycle's engine.
- In the main method, we create instances of Car and Motorcycle. We then call the startEngine() and stopEngine() methods on each object to start and stop the engines for both vehicle types.
Flowchart:
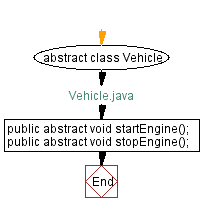
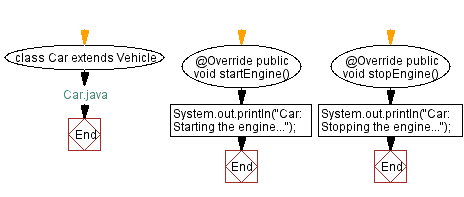
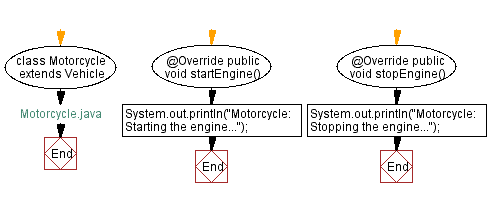
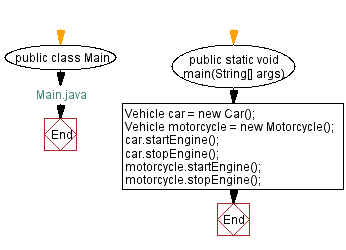
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract Shape3D Class with Sphere and Cube Subclasses.
Next: Java: Abstract Person Class with Athlete and LazyPerson Subclasses.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics