Java: Abstract Shape3D Class with Sphere and Cube Subclasses
Java Abstract Class: Exercise-6 with Solution
Write a Java program to create an abstract class Shape3D with abstract methods calculateVolume() and calculateSurfaceArea(). Create subclasses Sphere and Cube that extend the Shape3D class and implement the respective methods to calculate the volume and surface area of each shape.
Sample Solution:
Java Code:
// Shape3D.java
// Abstract class Shape3D
abstract class Shape3D {
// Abstract method to calculate volume
public abstract double calculateVolume();
// Abstract method to calculate surface area
public abstract double calculateSurfaceArea();
}
// Sphere.java
// Class Sphere extending Shape3D
class Sphere extends Shape3D {
// Private variable to store radius
private double radius;
// Constructor to initialize radius
public Sphere(double radius) {
// Setting the radius value
this.radius = radius;
}
// Overriding the calculateVolume method
@Override
public double calculateVolume() {
// Calculating the volume of the sphere using the formula (4/3) * π * radius^3
return (4.0 / 3.0) * Math.PI * Math.pow(radius, 3);
}
// Overriding the calculateSurfaceArea method
@Override
public double calculateSurfaceArea() {
// Calculating the surface area of the sphere using the formula 4 * π * radius^2
return 4 * Math.PI * Math.pow(radius, 2);
}
}
// Cube.java
// Class Cube extending Shape3D
class Cube extends Shape3D {
// Private variable to store side length
private double sideLength;
// Constructor to initialize side length
public Cube(double sideLength) {
// Setting the side length value
this.sideLength = sideLength;
}
// Overriding the calculateVolume method
@Override
public double calculateVolume() {
// Calculating the volume of the cube using the formula sideLength^3
return Math.pow(sideLength, 3);
}
// Overriding the calculateSurfaceArea method
@Override
public double calculateSurfaceArea() {
// Calculating the surface area of the cube using the formula 6 * sideLength^2
return 6 * Math.pow(sideLength, 2);
}
}
// Main.java
// Public class Main
public class Main {
// Main method
public static void main(String[] args) {
// Creating an instance of Sphere with radius 7.0
Shape3D sphere = new Sphere(7.0);
// Creating an instance of Cube with side length 6.0
Shape3D cube = new Cube(6.0);
// Printing the volume of the sphere
System.out.println("Sphere Volume: " + sphere.calculateVolume());
// Printing the surface area of the sphere
System.out.println("Sphere Surface Area: " + sphere.calculateSurfaceArea());
// Printing the volume of the cube
System.out.println("Cube Volume: " + cube.calculateVolume());
// Printing the surface area of the cube
System.out.println("Cube Surface Area: " + cube.calculateSurfaceArea());
}
}
Output:
Sphere Volume: 1436.7550402417319 Sphere Surface Area: 615.7521601035994 Cube Volume: 216.0 Cube Surface Area: 216.0
Explanation:
In the above exercise -
- The abstract class "Shape3D" has two methods calculateVolume() and calculateSurfaceArea(). The subclasses 'Sphere' and 'Cube' extend the Shape3D class and provide their own implementations for these abstract methods.
- The "Sphere" class calculates the volume and surface area of a sphere using the given radius, while the Cube class calculates the volume and surface area of a cube using the given side length.
- In the main method, we create instances of Sphere and Cube. We then call the calculateVolume() and calculateSurfaceArea() methods on each object to calculate and display the respective volume and surface area.
Flowchart:
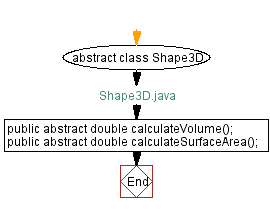
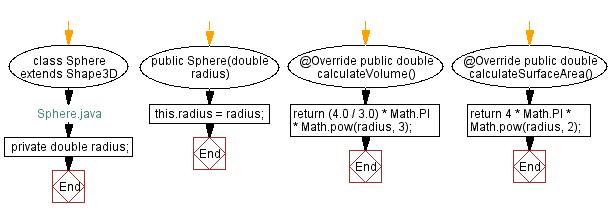
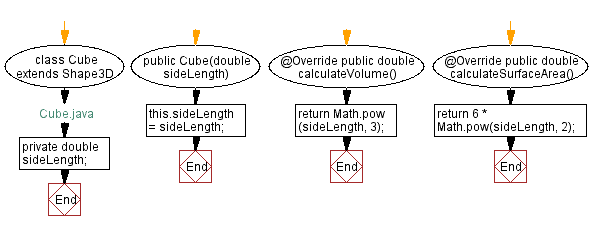
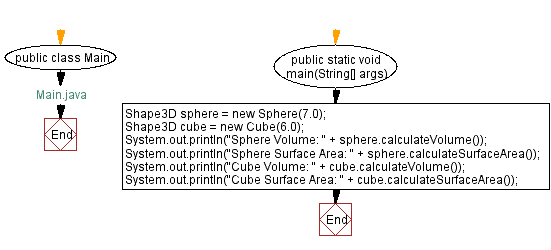
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract Employee class with Manager and Programmer subclasses.
Next: Java: Abstract Vehicle Class with Car and Motorcycle Subclasses.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics