Java: Abstract Employee class with Manager and Programmer subclasses
Java Abstract Class: Exercise-5 with Solution
Write a Java program to create an abstract class Employee with abstract methods calculateSalary() and displayInfo(). Create subclasses Manager and Programmer that extend the Employee class and implement the respective methods to calculate salary and display information for each role.
Sample Solution:
Java Code:
// Employee.java
// Abstract class Employee
// Define abstract class Employee
abstract class Employee {
// Declare protected variables for name and base salary
protected String name;
protected double baseSalary;
// Constructor to initialize name and base salary
public Employee(String name, double baseSalary) {
this.name = name; // Set the name
this.baseSalary = baseSalary; // Set the base salary
}
// Declare abstract method to calculate salary
public abstract double calculateSalary();
// Declare abstract method to display employee information
public abstract void displayInfo();
}
// Manager.java
// Subclass Manager
// Define class Manager that extends Employee
class Manager extends Employee {
// Declare private variable for bonus
private double bonus;
// Constructor to initialize name, base salary, and bonus
public Manager(String name, double baseSalary, double bonus) {
// Call superclass constructor to set name and base salary
super(name, baseSalary);
this.bonus = bonus; // Set the bonus
}
// Override the calculateSalary method from Employee
@Override
public double calculateSalary() {
// Return the total salary (base salary + bonus)
return baseSalary + bonus;
}
// Override the displayInfo method from Employee
@Override
public void displayInfo() {
// Print the manager's name
System.out.println("Manager Name: " + name);
// Print the base salary
System.out.println("Base Salary: $" + baseSalary);
// Print the bonus
System.out.println("Bonus: $" + bonus);
// Print the total salary
System.out.println("Total Salary: $" + calculateSalary());
}
}
// Programmer.java
// Class Programmer extending the Employee class
class Programmer extends Employee {
// Private variable to store overtime hours
private int overtimeHours;
// Private variable to store hourly rate
private double hourlyRate;
// Constructor to initialize name, baseSalary, overtimeHours, and hourlyRate
public Programmer(String name, double baseSalary, int overtimeHours, double hourlyRate) {
// Calling the constructor of the superclass (Employee)
super(name, baseSalary);
// Setting the overtime hours
this.overtimeHours = overtimeHours;
// Setting the hourly rate
this.hourlyRate = hourlyRate;
}
// Overriding the calculateSalary method
@Override
public double calculateSalary() {
// Calculating the total salary by adding baseSalary and the product of overtimeHours and hourlyRate
return baseSalary + (overtimeHours * hourlyRate);
}
// Overriding the displayInfo method
@Override
public void displayInfo() {
// Printing the programmer's name
System.out.println("Programmer Name: " + name);
// Printing the base salary
System.out.println("Base Salary: $" + baseSalary);
// Printing the overtime hours
System.out.println("Overtime Hours: " + overtimeHours);
// Printing the hourly rate
System.out.println("Hourly Rate: $" + hourlyRate);
// Printing the total salary
System.out.println("Total Salary: $" + calculateSalary());
}
}
// Main.java
// Public class Main
public class Main {
// Main method
public static void main(String[] args) {
// Creating an instance of Manager with name "Corona Cadogan", baseSalary 6000, and bonus 1000
Employee manager = new Manager("Corona Cadogan", 6000, 1000);
// Creating an instance of Programmer with name "Antal Nuka", baseSalary 5000, overtimeHours 20, and hourlyRate 25.0
Employee programmer = new Programmer("Antal Nuka", 5000, 20, 25.0);
// Calling displayInfo method on manager instance
manager.displayInfo();
// Printing a separator line
System.out.println("---------------------");
// Calling displayInfo method on programmer instance
programmer.displayInfo();
}
}
Output:
Manager Name: Corona Cadogan Base Salary: $6000.0 Bonus: $1000.0 Total Salary: $7000.0 --------------------- Programmer Name: Antal Nuka Base Salary: $5000.0 Overtime Hours: 20 Hourly Rate: $25.0 Total Salary: $5500.0
Explanation:
In the above exercise -
- The abstract class "Employee" has two abstract methods: calculateSalary() and displayInfo(). The subclasses Manager and Programmer extend the Employee class and provide their own implementations of abstract methods.
- The "Manager" class calculates the total salary by adding the bonus to the base salary, while the "Programmer" class calculates the total salary based on the number of overtime hours and the hourly rate.
- In the main method, we create instances of Manager and Programmer, and then call the displayInfo() method on each object to display the information specific to each role.
Flowchart:
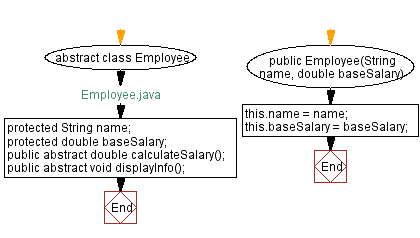
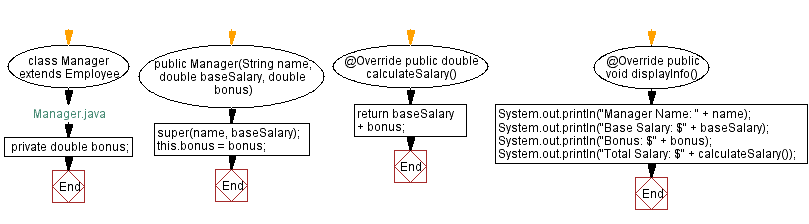
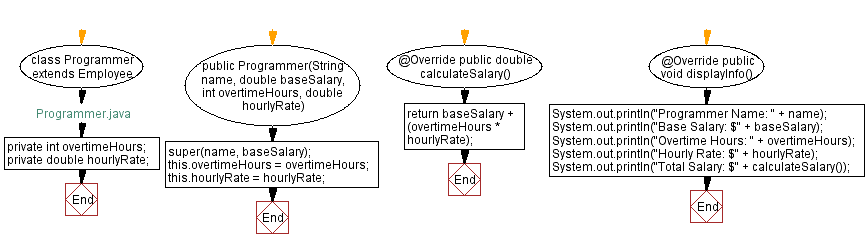
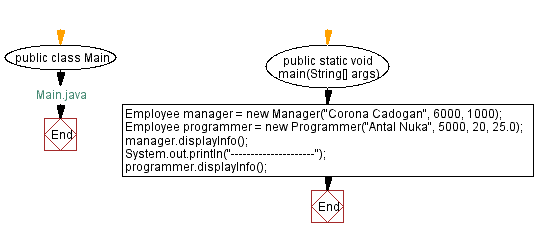
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract Animal Class with Lion, Tiger, and Deer Subclasses.
Next: Abstract Shape3D Class with Sphere and Cube Subclasses.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics