Java: Abstract Person Class with Athlete and LazyPerson Subclasses
Java Abstract Class: Exercise-8 with Solution
Write a Java program to create an abstract class Person with abstract methods eat() and exercise(). Create subclasses Athlete and LazyPerson that extend the Person class and implement the respective methods to describe how each person eats and exercises.
Sample Solution:
Java Code:
//Person.java
// Define an abstract class named Person
abstract class Person {
// Declare an abstract method eat
public abstract void eat();
// Declare an abstract method exercise
public abstract void exercise();
}
//Athlete.java
// Define a class named Athlete that extends the Person class
class Athlete extends Person {
// Override the eat method from the Person class
@Override
public void eat() {
// Print a message about the athlete's diet
System.out.println("Athlete: Include foods full of calcium, iron, potassium, and fiber.");
}
// Override the exercise method from the Person class
@Override
public void exercise() {
// Print a message about the athlete's training
System.out.println("Athlete: Training allows the body to gradually build up strength and endurance, improve skill levels and build motivation, ambition and confidence.");
}
}
//LazyPerson.java
// Define a class named LazyPerson that extends the Person class
class LazyPerson extends Person {
// Override the eat method from the Person class
@Override
public void eat() {
// Print a message about the lazy person's eating habits
System.out.println("Couch Potato: Eating while watching TV also prolongs the time period that we're eating.");
}
// Override the exercise method from the Person class
@Override
public void exercise() {
// Print a message about the lazy person's exercise habits
System.out.println("Couch Potato: Rarely exercising or being physically active.");
}
}
//Main.java
// Define the Main class
public class Main {
// The main method, the entry point of the program
public static void main(String[] args) {
// Create an instance of Athlete as a Person
Person athlete = new Athlete();
// Create an instance of LazyPerson as a Person
Person lazyPerson = new LazyPerson();
// Call the eat method on the athlete object
athlete.eat();
// Call the exercise method on the athlete object
athlete.exercise();
// Call the eat method on the lazyPerson object
lazyPerson.eat();
// Call the exercise method on the lazyPerson object
lazyPerson.exercise();
}
}
Output:
Athlete: Include foods full of calcium, iron, potassium, and fiber. Athlete: Training allows the body to gradually build up strength and endurance, improve skill levels and build motivation, ambition and confidence. Couch Potato: Eating while watching TV also prolongs the time period that we're eating. Couch Potato: Rarely exercising or being physically active.
Explanation:
In the above exercise -
- The abstract class "Person" with two abstract methods eat() and exercise(). The subclasses Athlete and CouchPotato extend the Person class and provide their own implementations for these abstract methods.
- The "Athlete" class describes how an athlete eats a balanced diet and engages in regular intense workouts, while the "LazyPerson" class describes how a couch potato consumes junk food and snacks while rarely exercising or being physically active.
- In the main method, we create instances of Athlete and LazyPerson, and then call the eat() and exercise() methods on each object to describe the eating and exercising habits of both types of people.
Flowchart:
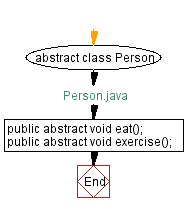
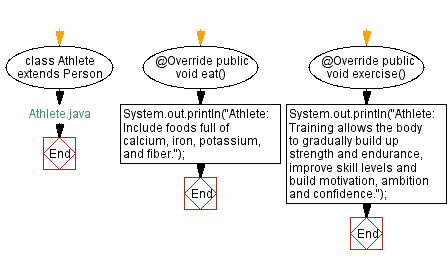
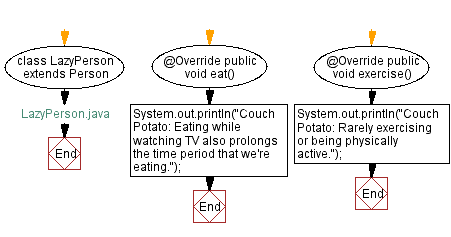
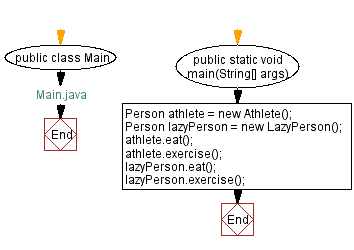
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Abstract Vehicle Class with Car and Motorcycle Subclasses.
Next: Abstract Instrument Class with Glockenspiel and Violin Subclasses.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics